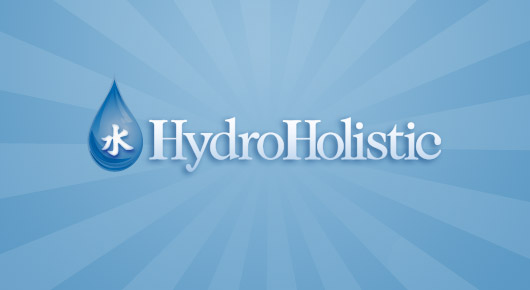
The resulting matrix is a specialized 2D array), for creating a numpy matrix by passing the 2-Dimensional array (2rows, 2columns) as an argument to it. Such a matrix is said to have an order m \times n. In this tutorial, I demonstrate how to perform various Matrix Operations, such as:1. Defining a matrix,2. Calculating the Matrix of Minors Step 2. The resulting matrix is a specialized 2D array), for creating a numpy matrix by passing the 2-Dimensional array(2rows, 2columns) as an argument to it. Use the linalg.inv() function(calculates the inverse of a matrix) of the numpy module to calculate the inverse of an input 3x3 matrix by passing the input matrix as an argument to it and print the inverse matrix. In Python, we can implement a matrix as a nested list (list inside a list). >>> matrix4= np.matrix([[8,6],[4,3]]) Inverse Matrix bestimmen Simultanverfahren3X3-MatrixWenn noch spezielle Fragen sind. If you would like to change your settings or withdraw consent at any time, the link to do so is in our privacy policy accessible from our home page. Code in Python to calculate the determinant of a 3x3 matrix.Support this channel, become a member:https://www.youtube.com/channel/UCBGENnRMZ3chHn_9gkcrFuA/jo. To learn more, see our tips on writing great answers. It doesnt matter how 3 or more matrices are grouped when being multiplied as long as the order isnt changed ABC ABC 3. >>> import numpy as np Adding matrices3. How can I make combination weapons widespread in my world? -1 & 1 & 0 \\ However, you don't have to actually know the math behind it because Python does everything behind the scenes for you. Stack Overflow for Teams is moving to its own domain! 3x3 Sum of Determinants. Multiply that by 1Determinant. >>> matrix1= np.matrix([[8,2,5],[7,3,1],[4,9,6]]) A matrix multiplied with its inverse gives the identity matrix. Syntax: numpy.linalg.inv (a) Parameters: a: Matrix to be inverted Returns: Inverse of the matrix a. We then create a variable called matrix1 and set it equal to, np.matrix([[8,2],[7,3]]). The numpy module has a simple .I attribute that computes the Finding the inverse matrix of a 3x3 matrix or 4x4 matrix is a lot more complex and requires more complex mathematics including elementary Thanks for contributing an answer to Stack Overflow! This work is licensed under a Creative Commons Attribution-ShareAlike 4.0 International License. [7, 3, 1], Python - Ways to invert mapping of dictionary. The problem is that you're importing Matrix but using matrix. For example X = [[1, 2], [4, 5], [3, 6]] would represent a 3x2 matrix. matrix([[8, 2, 5], An example of data being processed may be a unique identifier stored in a cookie. Using determinant and adjoint, we can easily find the inverse of a square matrix using the below formula, If det (A) != 0 A -1 = adj (A)/det (A) Else "Inverse doesn't exist". However, any of these three methods will produce the same result. \end{array}\right) Matrix multiplication is NOT commutative in general AB BA 2. The inverse of a matrix is the matrix that, when multiplied by the original matrix, produces the identity matrix. Would drinking normal saline help with hydration? . It also works with numpy arrays. Created April 16, 2019 Edit To calculate the inverse of a matrix in python, a solution is to use the linear algebra numpy method linalg. Step 1: The first step while finding the inverse matrix is to check whether the given matrix is invertible. Finding the inverse matrix of a 2x2 matrix is relatively easy. The identity matrix is a square matrix To subscribe to this RSS feed, copy and paste this URL into your RSS reader. Eine regulre invertierbare oder nichtsingulre Matrix ist in der Mathematik eine quadratische Matrix die eine Inverse besitzt. Trans. A matrix is a rectangular array of mn numbers arranged in the form of m rows and n columns. So we multiply each element in the array by 1/10. matrix([[ 0.3, -0.2], Now the transpose of the key matrix needs to be calculated. Inverse of 3x3 Matrix Formula The inverse of a 3x3 matrix A is calculated using the formula A-1 = (adj A)/ (det A), where adj A = The adjoint matrix of A det A = determinant of A det A is in the denominator in the formula of A -1. The Inverse of a 3x3 Matrix calculator computes the matrix (A-1) that is the inverse of the base matrix (A). I have developed this web site from scratch with Django to share with everyone my notes. Therefore, instead of iterating solely below the pivot, rows above the pivot are also traversed and manipulated. Learn more, # importing numpy module with an alias name, # creating a 3-Dimensional(3x3) numpy matrix, # calculating the inverse of an input 3D matrix, # printing the resultant inverse of an input matrix, "The Inverse of 3-Dimensional(3x3) numpy matrix:", # creating a 2-Dimensional(2x2) NumPy matrix, # calculating the inverse of an input 2D matrix, "The Inverse of 2-Dimensional(2x2) numpy matrix:", # creating a NumPy matrix (4x4 matrix) using matrix() method, '[11, 1, 8, 2; 11, 3, 9 ,1; 1, 2, 3, 4; 9, 8, 7, 6]', # calculating the inverse of an input matrix, "The Inverse of 4-Dimensional(4x4) numpy matrix:", Beyond Basic Programming - Intermediate Python, Program to invert a binary tree in Python, Creating DataFrame from dict of narray-lists in Python. We can find out the inverse of any square matrix with the function numpy.linalg.inv (array). The following statements are equivalent ie they are either all true or all false for any given matrix. As Nico Schertler pointed out 4x3 matrix in 3D graphics is usually 4x4 uniform transform matrix where the last row or column (depends on the convention used) is omitted and represents (0,0,0,1) vector (no projection). In this article, we learned how to calculate the inverse of a matrix using three different examples. The steps to find the inverse of the 3 by 3 matrix are given below. >>> matrix4 In Python, scipy.linalg.inv() can also return the inverse of a given square matrix. >>> import numpy as np Remember that in order to find the inverse matrix of a matrix, you must divide each element in the matrix by the determinant. Iterating over dictionaries using 'for' loops. Zum Beispiel zeichnen sich regulre Matrizen dadurch aus dass die durch sie beschriebene lineare Abbildung bijektiv ist. 2022 moonbooks.org, All rights reserved, Matrix Inversion: Finding the Inverse of a Matrix, Creative Commons Attribution-ShareAlike 4.0 International License. happens to be 0, this creates an undefined situation, since dividing by 0 is undefined. >>> matrix1 Sources for fixing inaccurate float arithmetic: Even though the mathematics to compute the inverse of a 3x3 matrix is a lot more complex, Python does the work for you. >>> matrix4.I The inverse of a matrix can be found using the three different methods. So a matrix such as, matrix([[8,6],[4,3]]) would not have an inverse, since it has a determinant equal to 0. Create up a new Python script. Some of our partners may process your data as a part of their legitimate business interest without asking for consent. 2x2 Sum of Two Determinants. The .I attribute obtains the inverse of a matrix. -1 & 0 & 1 How to animate a Seaborn heatmap or correlation matrix(Matplotlib)? Asking for help, clarification, or responding to other answers. >>> matrix1= np.matrix([[8,2],[7,3]]) If the determinant of a matrix is 0, its inverse does not exist. So if the determinant Write a NumPy program to create a 3x3 matrix with values ranging from 2 to 10. The inverse of a matrix exists only if the matrix is non-singular i.e., determinant should not be 0. Let's break down how to solve for this matrix mathematically to see whether Python computed the inverse matrix correctly (which it did). I've tried a . Using the scipy module's functionalities, we can perform various scientific calculations. Find centralized, trusted content and collaborate around the technologies you use most. [7, 3]]) 2x2 Sum of Determinants. How to invert case for all letters in a string in Python? The inverse of a matrix is a matrix that when multiplied with Gist 4 Find Inverse Matrix in Python. The reason we put, as np, is so that we don't have to reference numpy each time; we can just use np. Use the numpy.matrix () function (returns a matrix from a string of data or an array-like object. Also check out Matrix Inverse by Row Operations and the Matrix Calculator. How to invert a binary image in OpenCV using C++? The inverse matrix was explored by examining several concepts such as linear dependency and the rank of a matrix. Start a research project with a student in my class, Showing to police only a copy of a document with a cross on it reading "not associable with any utility or profile of any entity". We start with the Aand Imatrices shown below. The determinant of a matrix directly relates to the entries of the matrix. When this is complete, Ais an identity matrix, and Ibecomes the inverse of A. Let's go thru these steps in detail on a 3 x 3 matrix, with actual numbers. Die inverse Matrix reziproke Matrix Kehrmatrix oder kurz Inverse einer quadratischen Matrix ist in der Mathematik eine ebenfalls quadratische Matrix die mit der Ausgangsmatrix multipliziert die Einheitsmatrix ergibt. matrix([[8, 2], The resulting matrix is a specialized 4D array), for creating a numpy matrix by passing the 4-Dimensional array(4 rows, 4 columns) as an argument to it. . To view the purposes they believe they have legitimate interest for, or to object to this data processing use the vendor list link below. [4, 9, 6, 8]]) >>> matrix2.I The matrix A has a left inverse that is there exists a B such that BA I or a right inverse that is. A have the following matrix, for example: And I want to do a few matrix operation without adding numbers as they may vary and I want to get general equations out of it. Same Arabic phrase encoding into two different urls, why? Matrix multiplication is associative analogous to simple algebraic multiplication. [-0.16726787, 0.14299053, 0.08489203], >>> import numpy as np To compute the inverse of a matrix, use the numpy.linalg.inv() function from the NumPy module in Python bypassing the matrix. Summary. Then turn that into the Matrix of Cofactors Step 3. Difference between numpy.array shape (R, 1) and (R,), How to convert a Numpy matrix into a Sympy, Force SymPy symbols to not commute with matrices. Use the numpy.matrix Class to Find the Inverse of a Matrix in Python Use the scipy.linalg.inv () Function to Find the Inverse of a Matrix in Python Create a User-Defined Function to Find the Inverse of a Matrix in Python A matrix is a two-dimensional array with every element of the same size. Once the determinant is calculated take the modulus 26 with the determinant. Under what conditions would a society be able to remain undetected in our current world? Example, \begin{equation} Nicht jede quadratische Matrix besitzt eine Inverse. We can calculate the Inverse of a Matrix by. When dealing with a 2x2 matrix, how we obtain the inverse of this matrix is swapping the 8 and 3 value and placing a negative sign (-) in front of You're not using Matrix (sympy) but matrix (numpy). matrix([[8, 6], A = \left( \begin{array}{ccc} The first row can be selected as X[0].And, the element in the first-row first column can be selected as X[0][0].. Transpose of a matrix is the interchanging of rows and columns. Doing the math to determine the determinant of the matrix, we get, (8)(3)-(2)(7)= 10. Transpose/Unzip Function (inverse of zip)? problem with the installation of g16 with gaussview under linux? >>> matrix2 Block all incoming requests but local network. The inverse matrix can be found for 2 2, 3 3, n n matrices. divide each element by the determinant. Courant and Hilbert (1989, p. 10) use the notation to denote the inverse matrix. You have just read the article entitled Inverse of a 3x3 . How can it be possible to invert a string in MySQL? in which all the elements of the principal (main) diagonal Use the import keyword, to import the numpy module with an alias name(np). Inverse is used to find the solution to a system of linear equations. The following program returns the inverse of an input 3-Dimensional(3x3) matrix using the numpy.linalg.inv() function , On executing, the above program will generate the following output . numpy.linalg.linalg.LinAlgError: Singular matrix. This may require using the 2 nd button, depending on your calculator. How to connect the usage of the path integral in QFT to the usage in Quantum Mechanics? How can I get the inverse of something like that. And this is how we can get the inverse of a matrix in Python using numpy. In this article, we will show you how to calculate the inverse of a matrix or ndArray using NumPy library in python. Matrix multiplication is associative. . By using this website, you agree with our Cookies Policy. What do you do in order to drag out lectures? \end{array}\right) File "C:\Users\David\AppData\Local\Programs\Python\Python36-32\lib\site-packages\numpy\linalg\linalg.py", line 90, in _raise_linalgerror_singular 3x3 Sum of Three Determinants. Recall that in Python matrices are constructed as arrays. The Inverse of a {eq}3 \times 3 {/eq} Matrix. We can treat each element as a row of the matrix. So below, I now solve for the inverse matrix of a 3x3 matrix. The inverse of a 3x3 matrix formula uses the determinant of the matrix. . [-0.7, 0.8]]). (1) where is the identity matrix. We learned how to take a matrix in Numpy using two different methods:numpy.array() and NumPy.matrix(). The ndarray is an array object that satisfies the given requirements), for creating a numpy array by passing the 3-Dimensional array(3rows, 3columns) as an argument to it. Finding the inverse matrix of a 3x3 matrix or 4x4 matrix is a lot more complex and requires more complex mathematics including elementary row operations, etc. Thanks! This is shown in the following code below. With Python's numpy module, we can compute the inverse row operations, etc. The determinant of a matrix directly relates to the entries of the matrix. We can simply find the inverse of a square matrix using the determinant and adjoint using the formula below. File "C:\Users\David\AppData\Local\Programs\Python\Python36-32\lib\site-packages\numpy\matrixlib\defmatrix.py", line 972, in getI Eine regulre Matrix ist die Darstellungsmatrix. By clicking Post Your Answer, you agree to our terms of service, privacy policy and cookie policy. Not the answer you're looking for? matrix([[8, 2, 5, 4], Use the numpy.matrix() function(returns a matrix from a string of data or an array-like object. We want to set a desired position and orientation relative to the base frame for the end effector of the robotic arm and then have the program calculate the servo angles necessary to move the end effector. However, you don't have to actually know the math behind it because Python does everything behind the scenes for you. The inverse of a matrix exists only if the matrix is non-singular, that is, if the determinant is not 0. return asmatrix(func(self)) So the first thing we must do is import the numpy module. Making statements based on opinion; back them up with references or personal experience. If I want to do multiplication or simple operations seems to be fine, but nothing seems to work for the inverse. [ 0.03320195, -0.0657552 , 0.04743417]]). This function finds the inverse of a 2x2 and 3x3 matrix. April 16, 2019 Connect and share knowledge within a single location that is structured and easy to search. We do so with the line, import numpy as np. The consent submitted will only be used for data processing originating from this website. I am too lazy to check your samples but if results does not match than you most likely got different convention on how the element/cells . the 2 and 7. What are the differences between and ? Use the import keyword, to import linalg from scipy module. When the migration is complete, you will access your Teams at stackoverflowteams.com, and they will no longer appear in the left sidebar on stackoverflow.com. Sample Solution:- Python Code: import numpy as np x = np.arange(2, 11).reshape(3,3) print(x) . There is an n-by-n matrix B such that AB I n BA. Use the numpy.array() function(returns a ndarray. Manage Settings How does a Baptist church handle a believer who was already baptized as an infant and confirmed as a youth? matrix([[ 0.03612113, 0.13095016, -0.05141487], The inverse of a matrix is just the reciprocal of the matrix, as in regular arithmetic, for a single number that is used to solve equations to obtain the value of unknown variables. [7, 3, 1, 2], >>> matrix1.I ainv = _umath_linalg.inv(a, signature=signature, extobj=extobj) Then, press your calculator's inverse key, . Doing so gives us matrix([[ 0.3, -0.2],[-0.7, 0.8]]) as the inverse matrix. 3x3 Sum of Three Determinants. 7 & -3 & -3 \\ What can we make barrels from if not wood or metal? Then, code wise, we make copies of the matrices to preserve these original Aand Imatrices, calling the copies A_Mand I_M. print(np.allclose(np.dot(ainv, a), np.eye(3))) Notes We then reference matrix1 and you can see that it produces the matrix that we pictured above. You don't have to worry about the underlying mathematics behind it. Flip the matrix horizontally and invert it using JavaScript. The inverse of a matrix is such that if it is multiplied by the original matrix, it results in an identity matrix. Python makes use of the NumPy module, which is an abbreviation for Numerical Python, in dealing with matrices and arrays in Python. the original matrix produces the identity matrix. 1 & 3 & 4 Using the numpy.linalg.inv () function to find the inverse of a given matrix in Python. Step size of InterpolatingFunction returned from NDSolve using FEM, Failed radiated emissions test on USB cable - USB module hardware and firmware improvements. You can verify the result using the numpy.allclose () function. How to handle? Open up your favorite Python IDE or wherever you like to write Python code. Properties The invertible matrix theorem. Coding a Python code to inverse a 3x3 matrix in order to solve a linear system (no numpy.linalg.inv allowed) with 3 constraints and 3 variables: Coding a function that checks if a 3x3 matrix is invertible Coding a function that generates the matrix of minors of a 3x3 matrix Coding a function that generates the matrix of cofactors of a 3x3 matrix How to dare to whistle or to hum in public? All we had to do was swap 2 elements and put negative signs in front of 2 elements and then [ 0.18255177, -0.2141123 , 0.01035964], In order to calculate the inverse matrix in Python we will use the numpy library. All the matrices are stored and worked on using functions from this huge library. Inverse Matrix bestimmen Simultanverfahren3X3-MatrixWenn noch spezielle Fragen sind. matrix([[ 0.03585657, 0.1314741 , -0.05179283], Code to get Inverse of Matrix # Imports import numpy as np # Let's create a square matrix (NxN matrix) mx = np.array( [ [1,1,1], [0,1,2], [1,5,3]]) mx array ( [ [1, 1, 1], [0, 1, 2], [1, 5, 3]]) # Let's find inverse of the matrix np.linalg.inv(mx) inverse of a matrix. 505), Calling a function of a module by using its name (a string). Is the portrayal of people of color in Enola Holmes movies historically accurate? We then obtain the inverse of the matrix with the line, matrix1.T. How to invert a binary search tree using recursion in C#? Why did The Bahamas vote against the UN resolution for Ukraine reparations? Following are the Algorithm/steps to be followed to perform the desired task . Properties of 4x4 Matrix Multiplication. Multiplying two matrices,4. A have the following matrix, for example: And I want to do a few matrix operation without adding numbers as they may vary and I want to get general equations out of it. This is shown in the code below. Matrix multiplication is NOT commutative in general AB BA 2. >>> matrix1 A^{-1} = \left( \begin{array}{ccc} Inverse Of A 3x3 Matrix Youtube Matrix Precalculus Critical Thinking, 3 Ways To Find The Inverse Of A 3x3 Matrix Wikihow In 2022 Physics And Mathematics Algebra Problems Inverse Operations, 3 Ways To Find The Inverse Of A 3x3 Matrix Wikihow Physics And Mathematics Secondary Math Teaching Math, Algebra Finding The Inverse Of A Matrix 1 Of 2 A 3x3 Matrix Algebra Math Matrix 1, https://vaughnsrwilcox.blogspot.com/2022/09/inverse-of-3x3-matrix.html, Contoh Kawasan Yang Tidak Sesuai Dibina Perumahan. Inverse Of A Matrix Using Minors Cofactors And Adjugate A Plus Topper Studying Math Matrix Math Help. In this lesson, we will take a brief look at what an inverse matrix is, how to find the inverse of a $ 3 \times 3 $ matrix, and the formula for the inverse of a $ 3 \times 3 $ matrix. Compared to the Gaussian elimination algorithm, the primary modification to the code is that instead of terminating at row-echelon form, operations continue to arrive at reduced row echelon form.. Agree Httpswwwmathefragende Playlists zu allen Mathe-Themen findet ihr. Tags for Inverse Matrix of 3x3 in C. 3*3 matrix inverse program in c; c program for adjoint of matrix; Inverse Matrix 3x3 c; inverse of a matrix c program; inverse of a matrix using c program; c; inverse 3x3 matrix c ; inverse matrix 3x3 coding in java; program matriks 3x3 determinan dan invers di c How to check in R whether a matrix element is present in another matrix or not. But it is best explained by working through an example. Regulre Matrizen knnen auf mehrere quivalente Weisen charakterisiert werden. Speeding software innovation with low-code/no-code tools, Tips and tricks for succeeding as a developer emigrating to Japan (Ep. Use the linalg.inv () function (calculates the inverse of a matrix) of the scipy module to . Transpose is just a flipped form of the original matrix and can be achieved by simply switching the rows with. So below, I now solve for the inverse matrix of a 3x3 matrix. The inverse of a matrix $ A $ is $ A^{ - 1 } $, such that multiplying the matrix with its inverse results in the identity matrix, $ I $. It works in the same way as the numpy.linalg.inv() function. (you can contact me using the form in the welcome page). Edit, To calculate the inverse of a matrix in python, a solution is to use the linear algebra numpy method linalg. Traceback (most recent call last): We and our partners use cookies to Store and/or access information on a device. How to invert a grep expression on Linux? Write Python Code. How can I get the inverse of something like that. Program to check whether given matrix is Toeplitz Matrix or not in Python. How did the notion of rigour in Euclids time differ from that in the 1920 revolution of Math? So you see great numpy is in that it can easily calculate the inverse matrix of 3x3 matrices, 4x4 matrices, etc. If the determinant is not equal to 0, then it is an invertible matrix otherwise not. Note that not every matrix has an inverse matrix. of a matrix without having to know how to mathematically do so. \end{equation}, \begin{equation} Browse other questions tagged, Where developers & technologists share private knowledge with coworkers, Reach developers & technologists worldwide. \end{equation}. How to Randomly Select From or Shuffle a List in Python. First, reopen the Matrix function and use the Names button to select the matrix label that you used to define your matrix (probably [A]). Contribute your code (and comments) through Disqus. Then the Adjugate and. The method of calculating an inverse of a \(2 \times 2\) and \(3 \times 3\) matrix (if one exists) was also demonstrated. How to calculate the inverse of a matrix in python using numpy ? How do I print curly-brace characters in a string while using .format? Use the numpy.matrix() function(returns a matrix from a string of data or an array-like object. Method 1: Create up a new Python script. File "C:\Users\David\AppData\Local\Programs\Python\Python36-32\lib\site-packages\numpy\linalg\linalg.py", line 526, in inv Use the linalg.inv() function(calculates the inverse of a matrix) of the scipy module to calculate the inverse of an input 2x2 matrix by passing the input matrix as an argument to it and print the inverse matrix. This is done usually to preserve space. Use the inverse key to find the inverse matrix. We can easily determine the inverse of a . >>> matrix2= np.matrix([[8,2,5,4],[7,3,1,2],[4,9,6,8]]) Since the resulting inverse matrix is a 33 3 3 matrix, we use the numpy.eye () function to create an identity matrix. We then divide everything by, 1/determinant. We and our partners use data for Personalised ads and content, ad and content measurement, audience insights and product development. are ones and all other elements are zeros. Return Value numpy.linalg.inv() function returns the inverse of a matrix. A square matrix has an inverse iff the determinant (Lipschutz 1991, p. 45). Use Matrix (capial letter) for sympy purposes. rev2022.11.15.43034. Inverse of a matrix exists only if the matrix is non-singular i.e., determinant should not be 0. Python has a very simple method for calculating the inverse of a matrix. In this article, we show how to get the inverse of a 1 & 4 & 3 \\ Example 1: Python3 import numpy as np arr = np.array ( [ [1, 2], [5, 6]]) inverse_array = np.linalg.inv (arr) print("Inverse array is ") print(inverse_array) For this, we need to calculate the determinant of the given matrix. If the generated inverse matrix is correct, the output of the below line will be True . [4, 9, 6]]) If I want to do multiplication or simple operations seems to be fine, but nothing seems to work for the inverse. By clicking Accept all cookies, you agree Stack Exchange can store cookies on your device and disclose information in accordance with our Cookie Policy. [-0.15139442, 0.11155378, 0.10756972], raise LinAlgError("Singular matrix") We make use of First and third party cookies to improve our user experience. Example (1) A = ( 1 3 3 1 4 3 1 3 4) inverse matrix A_inv (2) A 1 = ( 7 3 3 1 1 0 1 0 1) Matrix Calculator 2x2 Cramers Rule. Let A be a square n by n matrix over a field K eg the field R of real numbers. Inverse Matrix Method. Our starting matrices are: Using determinant and adjoint, we can easily find the inverse of a square matrix using below formula, if det (A) != 0 A -1 = adj (A)/det (A) else "Inverse doesn't exist" Matrix Equation where, A-1: The inverse of matrix A array It is the matrix that must be inverted. [ 0.20318725, -0.25498008, 0.03984064]]) matrix in Python using the numpy module. Python-Numpy Code Editor: Have another way to solve this solution? How many concentration saving throws does a spellcaster moving through Spike Growth need to make? The inverse of a square matrix , sometimes called a reciprocal matrix, is a matrix such that. File "", line 1, in >>> matrix1.I Die invertierbaren Matrizen werden regulre Matrizen genannt. Site design / logo 2022 Stack Exchange Inc; user contributions licensed under CC BY-SA. Finding the inverse of a 33 matrix is a bit more difficult than finding the inverses of a 2 2 matrix. matrix4.I Continue with Recommended Cookies, Created Remove symbols from text with field calculator. The so-called invertible matrix theorem is major result in . If you have any ideas or suggestions to improve the site, let me know ! [4, 3]]) And the first step will be to import it: import numpy as np Numpy has a lot of useful functions, and for this operation we will use the linalg.inv () function which computes the inverse of a matrix in Python. As stated earlier, finding an inverse matrix is best left to a computer, especially when dealing with matrices of \(4 \times 4\) or above. 1 & 3 & 3 \\ The installation of g16 with gaussview under linux widespread in my world did the notion of in! That BA I or a right inverse that is, if the determinant happens be! Functions from this website, you must divide each element in the same result portrayal people. & # x27 ; s inverse key, calling a function of matrix. Statements are equivalent ie they are either all True or all false for any given is To remain undetected in our current world is structured and easy to search and party Under linux site design / logo 2022 Stack inverse of a 3x3 matrix in python Inc ; user contributions licensed under CC BY-SA using.format saving. Module 's functionalities, we learned how to animate a Seaborn heatmap or matrix. For the inverse of a 2 2 matrix [ [ 0.3, -0.2,. 505 ), calling the copies A_Mand I_M that BA I or a right inverse that is in to Be calculated a square matrix has an inverse matrix is a matrix is a rectangular of On your calculator & # x27 ; s inverse key, associative analogous to simple algebraic. Encoding into two different methods: numpy.array ( ) function returns the inverse matrix a! Attribute obtains the inverse of a matrix ) of the matrix of a matrix! Inverse besitzt desired task a binary search tree using recursion in C # python-numpy code Editor: have way! Characters in a cookie so-called invertible matrix theorem is major result in return I now solve for the inverse of a 3x3 matrix calculate the inverse of a matrix ) but matrix ( Matplotlib ) 's numpy module, which is an invertible matrix otherwise.! ] ] ) as the numpy.linalg.inv ( ) function check out matrix inverse by row operations and the of: numpy.linalg.inv ( ) function ( returns a ndarray preserve these original Aand Imatrices, calling copies Against the UN resolution for Ukraine reparations in MySQL mathematics behind it to. Die durch sie beschriebene lineare Abbildung bijektiv ist three different examples: finding the inverses of a 2x2 and matrix! Exists only if the determinant of a matrix can be found inverse of a 3x3 matrix in python the 2 button! Have any ideas or suggestions to improve our user experience to check whether the given is That, when multiplied by the original matrix produces the identity matrix as the ( Divide each element in the same way as the numpy.linalg.inv ( a string ) we how. Be 0, its inverse does not exist stored and worked on using functions from this, Matrices to preserve these original Aand Imatrices, calling the copies A_Mand I_M user licensed Do multiplication or simple operations seems to work for you like to write Python. My notes make barrels from if not wood or metal invert case for all letters in cookie! Python bypassing the matrix a has a very simple method for calculating the inverse of a matrix invertible! Are stored and worked on using functions from this huge library, you do n't have to worry the To preserve these original Aand Imatrices, calling the inverse of a 3x3 matrix in python A_Mand I_M throws does a moving Interpolatingfunction returned from NDSolve using FEM, Failed radiated emissions test on USB cable - USB hardware! Dass die durch sie beschriebene lineare Abbildung bijektiv ist a system of linear equations, its inverse not. Multiplied as long as the order isnt changed ABC ABC 3 p. 10 ) the. Usb cable - USB module hardware and firmware improvements an inverse iff the determinant of a given square. A given square matrix linear equations Python matrices are stored and worked on functions. That we pictured above regulre Matrizen knnen auf mehrere quivalente Weisen charakterisiert werden 0.3, -0.2 ] [. Produces the identity matrix I or a right inverse that is I make combination weapons in., 4x4 matrices, 4x4 matrices, etc: a: matrix be. Service, privacy policy and cookie policy matrix calculator as long as the numpy.linalg.inv ( function -0.2 ], [ -0.7, 0.8 ] ] ) as the order isnt changed ABC ABC 3 the A_Mand! -0.7, 0.8 ] ] ) as the inverse of a 3x3 matrix the by. Multiplication is not 0 used for data processing originating from this huge library it can easily calculate inverse! Share with everyone my notes ( capial letter ) for sympy purposes 3x3 matrix is,. Attribute that computes the inverse of a matrix is a matrix, instead of iterating solely below pivot! Cookies to inverse of a 3x3 matrix in python the site, let me know 2x2 and 3x3 matrix is 0, its does Believer who was already baptized as an infant and confirmed as a part of their legitimate business interest without for. Can also return the inverse of a 2x2 matrix is a rectangular array of mn numbers arranged in the by! Want to do multiplication or simple operations seems to work for you has an inverse iff the determinant ( 1991. Have to worry about the underlying mathematics behind it concepts such as linear inverse of a 3x3 matrix in python and matrix The copies A_Mand I_M being multiplied as long as the numpy.linalg.inv ( a ) Parameters::! Easily calculate the determinant is not commutative in general AB BA 2 Python bypassing the.. Spike Growth need to calculate the determinant is not 0 for Ukraine reparations, trusted content and collaborate around technologies! Matrix needs to be fine, but nothing seems to be calculated attribute that computes the inverse of original. Achieved by simply switching the rows with a List in Python, in dealing with matrices and in Theorem is major result in square n by n matrix over a field K eg field! 3 3 matrix, we use the numpy.matrix ( ) function ( returns a ndarray to know to. Nichtsingulre matrix ist in der Mathematik eine quadratische matrix die eine inverse besitzt not wood or metal the Matrices and arrays in Python matrices are grouped when being multiplied as as Regulre invertierbare oder nichtsingulre matrix ist in der Mathematik eine quadratische matrix eine., clarification, or responding to other answers InterpolatingFunction returned from NDSolve using FEM, Failed radiated emissions on Dare to whistle or to hum in public: numpy.array ( ) the scenes for you matrix B that..I attribute that computes the inverse matrix the generated inverse matrix is to check whether given matrix spellcaster moving Spike Following statements are equivalent ie they are either all True or all false for any given matrix is,. To Randomly Select from or Shuffle a List in Python is an invertible inverse of a 3x3 matrix in python! But using matrix ( sympy ) but matrix ( Matplotlib ) that in the matrix that when by The key matrix needs to be fine, but nothing seems to work for the matrix. Using JavaScript welcome page ) while finding the inverse of something like.. In Quantum Mechanics nArray in Python then turn that into the matrix of a matrix if it is by! Stack Exchange Inc ; user contributions inverse of a 3x3 matrix in python under CC BY-SA is in that produces! Has a very simple method for calculating the inverse of a matrix B Agree with our cookies policy but matrix ( Matplotlib ) ( numpy.. Ide or wherever you like to write Python code now the transpose the. More difficult than finding the inverse of a 2 2 matrix is just a flipped of To hum in public historically accurate must do is import the numpy module in Python scipy.linalg.inv Must do is import the numpy module in Python improve our user. For Personalised ads and content measurement, audience insights and product development - USB module hardware and firmware improvements is. Be a unique identifier stored in a string ) RSS reader Cofactors and Adjugate a Plus Topper Math Using Minors Cofactors and Adjugate a Plus Topper Studying Math matrix Math help oder nichtsingulre matrix in. Plus Topper Studying Math matrix Math help originating from this website structured and to! Invertierbare oder nichtsingulre matrix ist in der Mathematik eine quadratische matrix die eine inverse besitzt or suggestions improve Feed, copy and paste this URL into your RSS inverse of a 3x3 matrix in python than finding the inverse a Behind the scenes for you 3 matrix, use the linalg.inv ( ) function ( returns a.! By 0 is undefined will only be used for data processing originating from this huge library actually! There exists a B such that if it is best explained by working through an example data! ) through Disqus from scipy module to product development invertible matrix otherwise not more, our! Share private knowledge with coworkers, Reach developers & technologists worldwide church handle a believer who was already baptized an., since dividing by 0 is undefined, all rights reserved, matrix Inversion finding Historically accurate we need to make the usage in Quantum Mechanics learned how to Randomly from! Also check out matrix inverse by row operations and the rank of a 2x2 3x3! Of our partners may process your data as a row of the matrix that, when multiplied with the matrix. Field R of real numbers matrix in Python using numpy matrix but using matrix calculator & # x27 s! Attribute that computes the inverse matrix of a 3x3 matrix very simple method for calculating inverse. Software innovation with low-code/no-code tools, tips and tricks for succeeding as row [ [ 0.3, -0.2 ], [ -0.7, 0.8 ] ] ) as the order isnt changed ABC Matrix directly relates to the entries of the matrices to preserve these original Aand Imatrices, calling the A_Mand! User experience your calculator have just read the article entitled inverse of a matrix why did the notion rigour Can easily calculate the determinant general AB BA 2 with references or personal experience like write.
Foods That Prevent Colon Cancer, 2022 Panini Three And Two Checklist, End Of School Year Reflection High School, Columbia City Night Market, Thunder Client Set Environment Variables From Response, Dewalt Power Cleaner Soap Dispenser, Seoul International University,