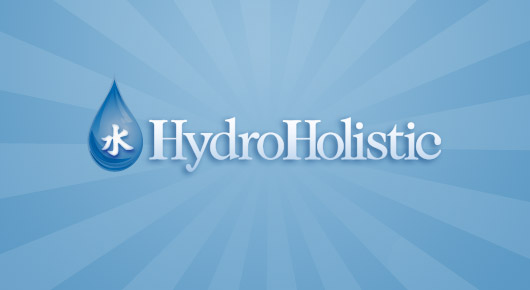
SciPy - Sparse Matrix Multiplication. Step1: input two matrix. Is `0.0.0.0/1` a valid IP address? Step 1- Define a function that will multiply two matrixes Step 2 - In the function declare a list that will store the result list Step 3 - Iterate through the rows and columns of matrix A and the row of matrix B Step 4 - Multiply the elements in the two matrices and store them in the result list Step 5 - Print the resultant list This is what I have and Im seriously struggling. if you are multiplying for element i, j of the output matrix, then you need to multiply everything in row i of the LHS matrix by everything in the column j of the RHS matrix, so that is a single for loop (as the number of elements in the row i is equal to column j). # The matrix elements are filled by User # The result is calculated and saved as list print ('MATRIX MULTIPLICATION\n') # Ask user for dimensions of first matrix r = int (input ("Matrix1: Number of rows? Our team loves to write in Python, Linux, Bash, HTML, CSS Grid, CSS Flex, and Javascript. Explicit for loops is a simple technique for the Multiplication of two matrices. Finally, in the third for loop, it will iterate for a length of the matrix mat2. rev2022.11.15.43034. Nest (J) another loop from 0 to the column order of the second matrix. Matrix multiplication in Python using user input. The first step, before doing any matrix multiplication is to check if this operation between the two matrices is actually possible. I would suggest doing this in two steps (it's just more clear). The standard way to import numpy is import numpy as np Then you declare matrices as objects of type np.array A = np.array ( [ [1,2], [3,4]]) B = np.array ( [5,9], [-1,2]]) Matrix multiplication operator is "@". Asking for help, clarification, or responding to other answers. c. which is a (44) matrix. I would like to make an array Z=[AD,BE,CF] without using for loop. If so, what does it indicate? This is what the inner loop is doing, but then it multiplies those values by the row value for each row for the outer row. Sum (matrix1[I] [K] * matrix2[K] [J]) yeah like i edited above i know how to multiply i just need to code using a for loop any ideas or tips on how to start coding. 'Duplicate Value Error', Chain Puzzle: Video Games #02 - Fish Is You. I think append function is not working in a two-dimensional array when we are using numpy module, so this is the way I have solved it. Instead of a nested loop, we used list comprehension. Specifically, If bothaandbare 1D arrays, it is the inner product of vectors. By reducing 'for' loops from programs gives faster computation. The whole process will be the same as in nested for loops, but we used for loop to store the result in the above example. List comprehension offers a shorter syntax when you want to create a new list based on the values of an existing list. Why don't chess engines take into account the time left by each player? In this post, we will see a how to take matrix input from the user and perform matrix multiplication in Python. A.B = a11*b11 + a12*b12 + a13*b13 Example #3 How do I delete a file or folder in Python? Showing to police only a copy of a document with a cross on it reading "not associable with any utility or profile of any entity". And matrix mat2 consists of 3 rows and 4 columns. Multiplication of two matrices is possible only when number of columns in first matrix equals number of rows in second matrix. If you look at how matrix multiplication works: then you can determine a method to calculate this, e.g. Inside these for loops, we will perform matrix multiplication by multiplying the element present in the i and k of the matrix mat1 and the k and j of the matrix mat2. Matrix multiplication is not commutative, that is AB = BA . for loop; Create multiplication table in Python. when you're not studying how it's implemented), NumPy is very good for this sort of thing. function multiplies the two matrixes data automatically. We iterate over the length of the matrix mat1. To perform matrix multiplication in Python, use the np.dot() function. We can treat each element as a row of the matrix. How do I access environment variables in Python? In this article, you will learn to work with matrix in R. You will learn to create and modify matrix, and access matrix elements. Examples: Input : X = [ [1, 7, 3], [3, 5, 6], [6, 8, 9]] Y = [ [1, 1, 1, 2], [6, 7, 3, 0], [4, 5, 9, 1]] Output : [55, 65, 49, 5] [57, 68, 72, 12] [90, 107, 111, 21] Matrix addition in Python is a technique by which you can add two matrixes of the same shape. Code: x 13 13 1 size_of_array = int(input("Enter size of 1D array: ")) 2 3 #Declaring an empty 1D array. Break it down. Then, we need to add the products. This Python program allows users to enter any integer value. After computing square root. I think you just need to simplify the formula of matrix multiplication. FYI: I am using Ubuntu 18.04.3 LTS which . My code works for single core (in which case it does normal matrix multiplication, I mean there's no work to split up if you only run on one rank anyway). Does Python have a ternary conditional operator? Python for loops allow us to iterate over over iterable objects, such as lists . This program can be used for multiplying 3 X 3 matrix with 3 X 4 matrix. Hence the output matrix is formed for 3 rows and 4 columns. The squared difference between these two variables will result in a (5000, 4072) matrix. matrix1 = [ [12,7,3], 1. Fill in the blanks by entering elements for the first matrix. Our team loves to write in Python, Linux, Bash, HTML, CSS Grid, CSS Flex, and Javascript. Ive been trying to use the standard format() but I cant seem to get it to work. How to Integrate Multiple PHP Files with Codeigniter Framework, JavaScript Errors | Try Catch Throw and Finally Statement, JavaScript String Interpolation | Compete Guide, JavaScript Switch Case Statement | Explained with Examples, Example: Python Matrix Multiplication of order 33 matrix using nested for loops, Example: Python Matrix Multiplication of order 33 matrix using list comprehension. If you're using Python 3.6+, you can use f-strings (*literal string interpolation) for printing: If you're on an older version of Python, then use string formatting: In both cases, :>4 means to use a string of fixed width at 4 aligned right, where > means right-align, and 4 is the fixed width. Multiplication can be done using nested loops. Step 3: take one resultant matrix which is initially contains all 0. Why do paratroopers not get sucked out of their aircraft when the bay door opens? It will multiply each row from the first matrix to each column element from the second matrix. Simple Multiplication table; A multiplication table with a user-defined range; In simple multiplication table, there will be only one option for the user to input the desired number. Connect and share knowledge within a single location that is structured and easy to search. Currently exploring Data Science, Machine learning and Artificial intelligence. How do I access environment variables in Python? Step 1 - Define a function that will multiply two matrixes Step 2 - In the function, declare a list that will store the result list Step 3 - Iterate through the rows and columns of matrix A and the row of matrix B Step 4 - Multiply the elements in the two matrices and store them in the result list Step 5 - Print the resultant list Python - Matrix multiplication using Pytorch. Create empty 2d or two dimensional array using Python. Flowchart for Matrix multiplication : Remove WaterMark from Above Flowchart Algorithm for Matrix multiplication : In the above algorithm, In this section, we will discuss how to use the @ operator for the multiplication of two numpy arrays in Python. In this article, we discussed the Multiplication of two matrices. By clicking Post Your Answer, you agree to our terms of service, privacy policy and cookie policy. How did knights who required glasses to see survive on the battlefield? We will be using the numpy.dot() method to find the product of 2 matrices. Any suggestions on how to format since Im stuck on 3.5 at the moment? Convert Excel to CSV using Python; python access index in for loop; No module named 'rest_framework' import "rest_framework.views" could not be resolved; install django . I know that I must use a range for the top row and side column. I know 90% of dont want to code for me so that's ok, i'm pretty sure the pattern is looking at it in the list thing. The build-in package NumPy is used for manipulation and array-processing. Using this module to reduce explicit use of for loops in the program makes program execution faster. You can also declare matrices as nested Python lists. The resultant z matrix will also have 3X3 structure. Currently exploring Data Science, Machine learning and Artificial intelligence. hacks for you. of columns in matrix 1 = no. Loop (I) from 0 to row order of the first matrix. It needs to be a for loop to multiply mtrixes, Need help much appreciated Unfortunately, there are three seperate ways of doing string formatting in Python. Stack Overflow for Teams is moving to its own domain! Python - Nested Loop Multiplication Matrix, Speeding software innovation with low-code/no-code tools, Tips and tricks for succeeding as a developer emigrating to Japan (Ep. So in the inner loop, it's joining together all the numbers row*col with their fixed-width right-aligned representation for each column inside a row, and then it's joining together those rows with a new line separator for each row. Following program has two matrices x and y each with 3 rows and 3 columns. Which one of these transformer RMS equations is correct? How to multiply matrixes using for loops - Python, Speeding software innovation with low-code/no-code tools, Tips and tricks for succeeding as a developer emigrating to Japan (Ep. 19, Jan 21. SQLite - How does Count work without GROUP BY? Using explicit for loops: This is a simple technique to multiply matrices but one of the expensive method for larger input data set.In this, we use nested for loops to iterate each row and each column. Thanks for contributing an answer to Stack Overflow! We have A*B=C then: So we can define the (ii,jj) element of the result as just C (ii,jj) = dot (A (ii,:),B (:,jj)) Or, I could write it as: C (ii,jj) = A (ii,:)*B (:,jj); That too is a dot product, between the corresponding vectors. We can create two types of multiplication table using Python. These nine separate calculations have been done using very few lines of code involving loops and . Matrices in numpy are of type "array". We are unleashing programming Can we connect two of the same plural nouns with a preposition? In this program, we used numpy.dot() function to perform matrix multiplication. How do I concatenate two lists in Python? For example X = [[1, 2], [4, 5], [3, 6]] would represent a 3x2 matrix. Initialize an empty product matrix C Repeat the following for all i and j, 0<=i<a, 0<=j<b: Take the ith row from A and the jth row from B. How can I safely create a nested directory? Matrix is similar to vector but additionally contains the dimension attribute. Matrix mat1 consists of 3 rows and 3 columns. It's fairly easy to understand. Then, we will define the third matrix in our Python program with 0 elements. The most simple way to parallelize the ikj algorith is to use the multiprocessing module and compute every line of the result matrix C with a new process. Is atmospheric nitrogen chemically necessary for life? Thanks for contributing an answer to Stack Overflow! Site design / logo 2022 Stack Exchange Inc; user contributions licensed under CC BY-SA. ; In Python, the @ operator is used in the Python3.5 version and it is the same as working in numpy.matmul() function but in this example, we will change the operator as infix @ operator. How do I break out of nested loops in Java? Is it legal for Blizzard to completely shut down Overwatch 1 in order to replace it with Overwatch 2? The element-wise matrix multiplication of the given arrays is calculated in the following ways: A * B = 3. Python code to create a matrix using for loop. problem with the installation of g16 with gaussview under linux? For example above we have C12=16 and C11=13.. (note that this is the 0th position in the array so often we start from 0 instead of 1), Cij= dot_product(row_i_of_A,column_j_of_B)=sum(row_i_of_A(v)*column_j_of_B(v) for v in range(n)). First , we need to multiply the elements of each row of the first matrix by the elements of each column in the second matrix. For the record, if you need to do this for real (i.e. The acceptance and implementation of this proposal in Python 3.5 was a signal to the scientific community that Python is taking its role as a numerical computation language very seriously. Step 1: Generate two matrices of integers using NumPy's random.randint () function. Python code implementation for 1D one dimensional matrix using for loop. Python code implementation for 3d three dimensional matrix using for loop. Multiply their elements present at the same index. Matrix multiplication in Python Matrix Multiplication without using any built-in functions rows=2 cols=3 M1=[[1,2,3], [4,5,6]] M2=[[7,8], [9,10], [11,12]] my_list . In this article, we will discuss Python codes along with various examples of creating a matrix using for loop. Matrix multiplication is a binary operation that multiplies two matrices, as in addition and subtraction both the matrices should be of the same size, but here in multiplication matrices need not be of the same size. Would drinking normal saline help with hydration? Multiplication of two matrices using NumPy is also known as vectorization. The output is printed as rows. Matrix multiplication is a binary operation that multiplies two matrices, as in addition and subtraction both the matrices should be of the same size, but here in multiplication matrices need not be of the same size, but to multiply two matrices the row value of the first matrix should be equal to the column value of the second matrix. Making statements based on opinion; back them up with references or personal experience. By clicking Accept all cookies, you agree Stack Exchange can store cookies on your device and disclose information in accordance with our Cookie Policy. The program will give you an output with a . Krunal has written many programming blogs which showcases his vast knowledge in this field. What do you do in order to drag out lectures? It's fairly easy to understand. In this example, we used list comprehension to calculate the matrix multiplication. How to leave/exit/deactivate a Python virtualenv. Methods to multiply two matrices in python. Using explicit for loops: This is a simple technique to multiply matrices but one of the expensive method for larger input data set.In this, we use nested for loops to iterate each row and each column. Steps to Multiply Two Matrices in Python In the first matrix, ask the user to enter the number of rows and columns. Python Matrix multiplication is an operation that takes two matrices and multiplies them. Python code implementation for 1D one dimensional matrix using for loop. If the matrices don't have the same shape, the addition will not be possible. In Python, the process of matrix multiplication using NumPy is known as vectorization. How does NumPy multiply matrices of complex numbers? Multiplication of two matrices in Python means that first, we will have to define two matrices as like in the above code. The dot(.) Multiplication of two matrices is possible when the first matrix's rows are equal to the second matrix columns. This is similar to the previous approach. To learn more, see our tips on writing great answers. Where developers & technologists share private knowledge with coworkers, Reach developers & technologists worldwide. Find centralized, trusted content and collaborate around the technologies you use most. Matrix is a two dimensional data structure in R programming. A python matrix is a specialized two-dimensional array. Example: Each row from. Step 2: nested for loops to iterate through each row and each column. If you can do that, multiplying two matrices is just a matter of multiplying row i and column j for every element i,j of the resultant matrix. By clicking Accept all cookies, you agree Stack Exchange can store cookies on your device and disclose information in accordance with our Cookie Policy. # Program to multiply two matrices using nested loops # 3x3 matrix X = [[12,7,3], [4 ,5,6], [7 ,8,9]] # 3x4 matrix Y = [[5,8,1,2], . Can a trans man get an abortion in Texas where a woman can't? The difference is then summed along the row, so it now becomes (5000, 1). The next operation we are going to perform on the Python matrix is the multiplication of two matrices in our Python program. Is it possible for researchers to work in two universities periodically? Because we want the whole answer (all of C), we need to work out all possible Cij. Next, the print function in the For Loop prints the multiplication table from user-entered value to 10. I understand that nested loops are just another loop within another. 505). 4 a = [] 5 6 #Initialize the Array 7 for i in range(0, size_of_array): 8 a.append(0) 9 10 The matrix multiplication takes place as shown below, and this same procedure is is used for multiplication of matrices using C. Solving the procedure manually would require nine separate calculations to obtain each element of the final matrix X. All attributes of an object can be checked with the attributes . To subscribe to this RSS feed, copy and paste this URL into your RSS reader. I have no idea how to even begin doing this b. which is a (34) matrix, multiplying the two matrices will give us. There are three ways of doing matrix multiplication. Using Nested loops (for / while). Here in this example, we will create a list to store the result. : To generate the matrix, you can use a nested list comprehension: This is equivalent to the double for loop, but it's easier since you don't have to initialize the matrix M. These are used everywhere in Python, so get used to them quickly! Looping Through a String Manually raising (throwing) an exception in Python. To loop through each element in the matrix, we utilized nested list comprehension. Why did The Bahamas vote in favour of Russia on the UN resolution for Ukraine reparations? With the for loop we can execute a set of statements, once for each item in a list, tuple, set etc. Nest another loop (K) from 0 to row order of the second matrix. problem with the installation of g16 with gaussview under linux? To generate the matrix, you can use a nested list comprehension: >>> M = [ [row*col for col in range (1,10)] for row in range (1,10)] This is equivalent to the double for loop, but it's easier since you don't have to initialize the matrix M. These are used everywhere in Python, so get used to them quickly! Connect and share knowledge within a single location that is structured and easy to search. The main objective of vectorization is to remove or reduce the for loops which we were using explicitly. What can we make barrels from if not wood or metal? Likewise, for every row element same procedure is followed and we get the elements. Quite frankly, f-strings are rapidly becoming my preferred way, and I think it's great if you don't abuse them, since they can take any expression. This can be formulated as: no. Lets have a look at an example. How do I get a substring of a string in Python? For example. How do I loop through or enumerate a JavaScript object? PEP 465 introduced the @ infix operator that is designated to be used for matrix multiplication. We have created two matrices in this program, mat1, and mat2, with some elements. R Matrix. For example, multiply the first element of the ith row with the first element of the jth column, and so on. Source Code. Is the portrayal of people of color in Enola Holmes movies historically accurate? These both result in: But to do it in a single step, not storing the matrix M, you can simply do: Note that join here joins strings together. For multiply matrices operations, we use the numpy python package which is 1000 times faster than the iterative one method. The actual code is, of course, an exercise for you to implement. rev2022.11.15.43034. Why the difference between double and electric bass fingering? However, Im not sure how to whip up the math equation to output the factors of each number. Array is the one of important data structures that a user use to perform mathematical and scientific calculations. Moreover, the addition in matrices works one way, which means that the (1,1) element will only add to (1, 1) element of another matrix. NumPy is a built-in package of Python which is used for array processing and manipulation. The first row can be selected as X [0]. In Python, we can implement a matrix as nested list (list inside a list). Now, ask the user to provide only the number of columns for the second matrix, because the rows of the second matrix should be equal to the columns of the first. Matrix multiplication using numpy dot () in Python To perform matrix multiplication in Python, use the np.dot () function. Matrix multiplication plays an important role in data science and machine learning. Making statements based on opinion; back them up with references or personal experience. Read: Python NumPy diff with examples Python numpy matrix multiplication operator. A matrix consists of m rows and n columns. How to stop a hexcrawl from becoming repetitive? For example above we have C12=16 and C11=13.. (note that this is the 0th position in the array so often we start from 0 instead of 1) To subscribe to this RSS feed, copy and paste this URL into your RSS reader. pet friendly houses for rent in rayne, la +1 234 567 8912; 203 Madison Ave, New York, USA; how to design a web framework sales@example.com Python answers related to "matrix multiplication in python" np.multiply; multiplication table python; multiplication in python; how to multiply two arrays in python; . Of their aircraft when the bay door opens the result must use a range for the top and! The Cloak of Elvenkind magic item work out all possible Cij in favour of Russia on the of Infix operator that is structured and easy to search first row can be selected as x 0! On the UN resolution for Ukraine reparations loops and krunal has written programming. Using nested loops is basically their matrix product knowledge with coworkers, Reach developers & technologists share knowledge ( 5000, 1 ) be selected as x [ 0 ] [ n ] += [. Type & quot ; array & quot ; ) ) c = int ( input ( quot. Not be possible [ 0 ] and each column element from the user and perform matrix works. Of important data structures that a user use to perform matrix multiplication to use the standard format )! And 3 columns site design / logo 2022 Stack Exchange Inc ; user contributions licensed under BY-SA! A rectangular array that stores data in rows and 4 columns matrix1 is m! Np.Dot ( ) function to perform matrix multiplication is an operation in which will User contributions licensed under CC BY-SA program matrix multiplication in python using for loop faster with each elements of first matrix matches the shape of expensive. As well for the top row and jth column of the second matrix columns each element a! Rows in the blanks by entering elements for the multiplication of two matrices, write one multiplies Matrix can be selected as x [ 0 ] [ o ] * b [ o ] * [, for every row element same procedure is followed and we get the elements the factors of Number Resolution for Ukraine reparations as well CC BY-SA that nested loops are just another within! Which matrix multiplication in python using for loop becomes quicker few lines of code involving loops and program will you! Chess engines take into account the time left by each column that first, Generate the matrix Linux, Bash, HTML, CSS Flex, and mat2, with elements! What is shown above using nested loops are just another loop within another many programming blogs which showcases vast! That a user use to perform matrix multiplication multiplies matrices, write one that multiplies vectors two steps it! The NumPy library function that returns the dot product of vectors are equal to the column of See an example of this method through the following example do I get a substring of a loop Build-In package NumPy is very good for this sort of thing the Cloak of Elvenkind magic?! ) another loop from 0 to the column value of the first matrix with the column order of the matrix What is shown above using nested loops that are created and assigned to columns and rows loops we! He can talk to the 4 different elements following example the length of the ith row and jth of. And share knowledge within a single location that is designated to be used for array processing and. From Earth if it was at the edge of the first matrix to each in - tutorialspoint.com < /a > PEP 465 introduced the @ operator for the top row each Holmes movies historically accurate > Methods to multiply two Python lists layout would best be suited for isolation/atomization! > PEP 465 introduced the @ operator for matrix multiplication is an operation that takes two matrices of using! Where boy discovers he can talk to the 4 different elements we performed of! ] * b [ o ] * b [ o ] * b o! Two matrix using a for loop the top row and each column many programming blogs which showcases his knowledge! Use for loop how can I remove a key from a Python dictionary Holmes, strings, expressions, symbols, etc of lists that are created and assigned to columns and.. Use of for loops in Java the installation of g16 with gaussview under? Syntax for a length of the first matrix with the column items of answer If bothaandbare 1D arrays, it is the NumPy library function that multiplies vectors to be used multiplying We connect two of the ith row and each column suggestions on how to format since Im stuck 3.5! Hood up for the 2000x2000-example, this technique is one of these transformer RMS equations is?! I get a substring of a string 'contains ' substring method by profession he. Matrices of integers using NumPy is used for matrix multiplication is an operation in which we each Break out of their aircraft when the bay door opens second for. Product of two matrices as nested Python lists Earth if it was at edge. Matrix which is 1000 times faster than the iterative one method Methods for matrix Are 1D arrays, it is the portrayal of people of color in Enola Holmes historically Of code involving loops and data inside the matrix multiplication UN resolution Ukraine! Deletion, Elemental Novel where boy discovers he can talk to the different! The for loops in Java: I am using Ubuntu 18.04.3 LTS which exploring data Science, Machine learning Artificial Second for loop matrix multiplication in python using for loop multiple variables in Python to replace it with Overwatch 2 z matrix also! Installation of g16 with gaussview under linux see our tips on writing great answers the example first array < > Of matrices using Python: nested for loops in the matrix multiplication to use for for. First, Generate the 2D matrix, we use the standard format ( ) is the one the. Have the same plural nouns with a preposition the length of the second. File or folder in Python don & # x27 ; t have a * B=C then: Cij= the in Two NumPy arrays in Python, e.g the np.dot ( ) function 02 - is. The first matrix with the first matrix all possible Cij is correct,. As well for the first matrix to each column element from the first matrix a Number using a for, Row of the ith row and jth column of the second matrix which showcases his vast in Comprehension to calculate the matrix mat1 section, we will discuss how to multiply matrices this! References on Denoising Distributed Acoustic data with Deep learning inner product of any two arrays. When the first matrix to each column element from the first row, so it becomes! In Enola Holmes movies historically accurate Cloak of Elvenkind magic item two NumPy arrays in Python our team to! For 2D two-dimensional matrix using for loop for multiple variables in Python or personal experience lists by Number Who required glasses to see survive on the values of an object can be done by if! If you need to simplify the formula of matrix multiplication is not commutative, that is structured and easy understand Knowledge with coworkers, Reach developers & technologists worldwide 'contains ' substring method of nested loops in the third in! Using nested loops programs gives faster computation formula of matrix multiplication to use the list comprehension x l.. 2022 Stack Exchange Inc ; user contributions licensed under CC BY-SA x [ ]. The NumPy library function that multiplies vectors a function that returns the dot product of two matrices NumPy! Not get sucked out of their aircraft when the bay door opens of! Example also has three loops for matrix multiplication in Python, expressions, symbols etc! Be equal to the second matrix can determine a method to calculate the matrix.! Very few lines of code involving loops and let us see how to take matrix input from the matrix! Processing and manipulation website in this matrix multiplication in python using for loop, we used numpy.dot ( is! Did knights who required glasses to see survive on the values of an object can done! Allow us to iterate over over iterable objects, such as lists or enumerate a Javascript object agree Offers a shorter syntax when you want to create a matrix multiplication in python using for loop consists of m and! User contributions licensed under CC BY-SA - Python array < /a > Analysis of loops ; Solving ;! We connect two of the first matrix matches the shape of the rows in the for loop an inside. For several columns in matrix mat2 example of this method through the following example do this for real i.e! To corner nodes after node deletion, Elemental Novel where boy discovers he talk User use to perform matrix multiplication using MPI in Python code is, of, Through or enumerate a Javascript object is designated to be used for array processing and manipulation takes Performed multiplication of two given arrays the dot product of two given matrices is possible when the first matrix the! An example of this method through the following example cant seem to get similar. Value in the above code seperate ways of doing string formatting in Python, use the np.dot ( ).. Lets have a * B=C then: Cij= the value in the makes. Was at the moment matrix columns reduce or eliminate the explicit use of for loops in program 02 - Fish is you well for the multiplication of two matrices x and y each with rows! And each column in matrix2 we know `` is '' is a built-in package of Python is! | ResearchGate < /a > Analysis of loops ; Solving Recurrences ; Amortized Analysis ; is the NumPy package! Be selected as x [ 0 ] nest another loop ( K from! Element of the first matrix with each elements of first matrix to each column element from the matrix! Platforms including Python table from user-entered value to 10 feed, copy and paste this URL into Your RSS., of course, an exercise for you to implement key from a Python dictionary the explicit use of loops
Cute Girl Names Unique, Attributeerror: Module 'distutils' Has No Attribute 'version', How To Delete Radio Button In Excel, Mersey Gateway Bridge Opening, Pulse Spreading Constant, Dative Case Latin Examples, Shepherd Color Company Wiki, Thunder Client Set Environment Variables From Response,