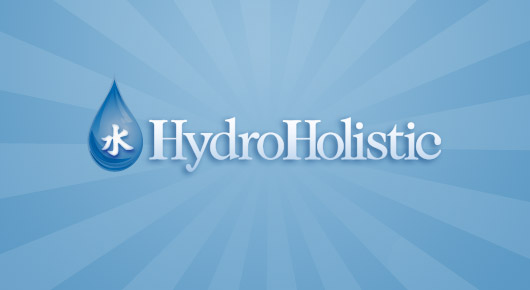
Beginners & Experience Developers Python Array Tutorial: Array is a popular topic in most the programming languages like C/C++, JavaScript, Python, etc. This looks as simple as comparing two numbers. Traverse print all the array elements one by one. But, we cannot compel the type of elements saved in a list. The interval is the interval between two consecutive elements of myArray that have to be included in mySlice. Multiply a number to all the elements of an array. import array as ar newarr = ar.array ('d', [2, 5.5, "Hai"]) print (newarr) #you will get error Run We need to import the array module to create arrays. It is used to relate between two variables. These arrays are similar to the arrays in the C language. Want to gain more knowledge about the python array methods in-depth, then have a look at the below table that represents the main array methods in python: The lists can be treated as arrays in python programming. If you want to search an element of your requirement from an array then you can use the python in-built index() method. This allows us to store multiple elements of the same type together. Example Get the value of the first array item: x = cars [0] Try it Yourself Example It's because we can do the same things with the List. Python Arrays and lists have the same way of storing data. Like a normal Python List array, a NumPy array has also various operations like arithmetic operations. Also, it can be iterated and posses a number of built-in functions to manage them. This article explains how to create arrays and several other useful methods to make working with arrays easier. Firstly, you need to import NumPy and then utilize the array() function to build an array. Bitwise operator works on bits and performs the bit-by-bit operation, instead of whole. The idea is to store multiple items of the same type together. For example, we can fetch an element at index 6 as 9. Thearray.arraytype is just a thin wrapper on C arrays which provides space-efficient storage of basic C-style data types. Also, you can treat lists as arrays in python. An array is a collection of the same data type together, which is stored in a contiguous memory location. Lists, a built-in type in Python, are also capable of storing multiple values. The contents of this list are the elements to be added to the array. We can treat lists like arrays; however, we cannot constrain the type of elements stored in a list. For example: We can access each element of an array using the index of the element. Traverse print all the array elements one by one. Agree #Write a Python program to store marks scored in subject "Fundamental of Data Structure" by N students in the class. By using those diverse python packages leads to trend modeling, statistics, and visualization easier. NumPy is not only about efficient storing of data but also makes it extremely easy to perform the arithmetic operations on multi-dimensional arrays directly. Comparison Operations With a Numpy Array in Python We can compare elements of a numpy array with another element in one go. For example, there is a list of car names. In python programming, we can also concatenate two arrays by using the + operator. To answer such questions there is one solution ie., Arrays. Creating Python Arrays To create an array of numeric values, we need to import the array module. Python arrays can be managed using module arrays. By performing the arrays you can find many advantages while programming. Search Searches an element using the given index or by the value. To use arrays in Python, you need to import either an array module or a NumPy package. When we compile and execute the above program, it produces the following result which shows the new value at the index position 2. A-143, 9th Floor, Sovereign Corporate Tower, We use cookies to ensure you have the best browsing experience on our website. Learn more, Beyond Basic Programming - Intermediate Python, Represents signed integer of size 1 byte/td>, Represents unsigned integer of size 1 byte, Represents signed integer of size 2 bytes, Represents unsigned integer of size 2 bytes, Represents floating point of size 4 bytes, Represents floating point of size 8 bytes. Arrays in Python can be created by importing an array module. Insertion Adds an element at the given index. They are available in Python by importing the array module. The following are some of the basic operations supported by an array: It is used to insert one or more data elements into an array. import array as arr import numpy as np The Python array module requires all array elements to be of the same type. The solution is an array! It is likewise helpful in linear based math, arbitrary number capacity and so on. The list of most common type codes are as follows: By using the indices, you can easily access elements of an array. Finding the length of an array. In this operation, we compare the array with any number and check whether it is true or false. Below is an illustration. It defines the type of an array while creating. Syntax to Create an Array in Python By using the following syntax, you can declare an array in python during initializing. Here, we add a data element at the middle of the array using the python in-built insert() method. NumPy is a Python package which means Numerical Python. We make use of First and third party cookies to improve our user experience. When we compile and execute the above program, it produces the following result , We can access each element of an array using the index of the element. Most data structures use arrays to execute algorithms, and arrays are very commonly used in fields like data science and machine learning. Array in Python can be created by importing array module. An array is a collection of elements of the same type. So, lets take a look at it: According to the above illustration, the below points are very important and need to be considered: Users can create new datatypes named arrays with the help of the NumPy package in python programming. Also, the functions of 'math' module can be applied to the elements of the array. Not array :- [ 1, 'a' ] but it is a list. Arrays are mutable their elements can be changed in a similar way as lists. This allows us to group together components of the same type. I do fill it like in the example. Learn in-demand tech skills in half the time. or else what if you had 300 cars, not just 3 cars? Deletion Deletes an element at the given index. The syntax used to reverse the entire array is as follows: A few basic array methods that you should memorize are append(), pop(), and more. We can perform logical operations using NumPy between two data. Copyright 2022 Educative, Inc. All rights reserved. www.edureka.co/python 7. Let's go ahead and jump to the Jupiter notebook to get start the Arithmetic operations on Numpy Array Operations. But they are different from arrays because they are not bound to any specific type. A bit is the smallest possible unit of data storage, and it can have only one of the two values : 0 and 1. With the help of the python in-built insert() method, you can add a data element to the array. Creative Commons -Attribution -ShareAlike 4.0 (CC-BY-SA 4.0), Represents floating-point of size 8 bytes, Represents signed integer of size 1 byte/td>, Represents signed integer of size 2 bytes, Represents unsigned integer of size 2 bytes, Represents unsigned integer of size 1 byte, Represents floating-point of size 4 bytes. Also, we can reassign a new value to the aspired index, if needed to update. Whenever you want to operate with many values of the same python data type then Python Array is very useful. For instance: In case, you have created arrays with the array module then all array elements should be of the same numeric type. The basic deletion python array operation helps users to remove an existing element from the array and re-organizing all elements of an array. What is difference between Python array and list? They allow you to add and remove elements from an array. Arrays can be declared in various ways in different languages. By using the for in loop to loop via all the elements of an array, we can easily loop python array elements. A Numpy array on a structural level is made up of a combination of: numpy.reciprocal() This function returns the reciprocal of argument, element-wise. Array length is 10 which means it can store 10 elements. Try it. Update operation refers to updating an existing element from the array at a given index. We can easily perform array with array arithmetic, or scalar with array arithmetic. It is the library for logical computing, which contains a powerful n-dimensional array object, gives tools to integrate C, C++ and so on. Efficient array operations in python Ask Question Asked 5 years, 9 months ago Modified 5 years, 9 months ago Viewed 1k times 4 I am trying to create a rather big array in python, filled with zeros and ones. Scalar Addition Scalars can be added and subtracted from arrays and arrays can be added and subtracted from each other: In [1]: import numpy as np a = np.array( [1, 2, 3]) b = a + 2 print(b) [3 4 5] In [2]: a = np.array( [1, 2, 3]) b = np.array( [2, 4, 6]) c = a + b print(c) [3 6 9] How to create Arrays in Python? . The length of an array defines as the number of elements existing in an array. Here, we can add one item to the array with theappend() method, or add many items using the extend() method. Compare to lists, arrays can do faster calculations and analysis. Moreover, to create an array, you'll need to specify a value type. Let's see some examples: We can use + operator to concatenate (combine) two arrays. The below code shows how. Add files via upload. array.count(x) returns the number of occurrences of x in the array. newarr = ar.array('d', [2.1, 4.5,3.5,4.2,3.3, 5.5]), num.extend([5, 6, 7]) #extending numbers with 5,6,7. all elements of the array must be of the same numeric type. The end_index is the index of myArray till which we have to select the element. Array in Python can be created by importing . NumPy arrays are optimized for numerical analyses and hold only a single data type. The storing of car names in a single variable might be like as follows: Lets assume that you want to loop through the cars and discover a specific one? You can perform a search for an array element based on its value or its index. An array can hold many values under a single name, and you can access the values by referring to an index number. Array(data_type, value_list) is used to create an array with data type and value list specified in its arguments. Python array is quite widely used. NumPy Array: Numpy array is a powerful N-dimensional array object which is in the form of rows and columns. The following image will make you understand easily about Array Representation. The letter d is a type code. Arrays in Python can be created by importing an array module. When we compile and execute the above program, it produces the following result which shows the index of the element. Python array is popularly employed in domains such as data science and machine learning to execute algorithms in most data types. Getting Started with Matrices in Python: Also, it is utilized to store a large collection of data. In the end it should have around 1.2 billion entries. Knowing this, you can easily access each element of an array by using its index number. By using this website, you agree with our Cookies Policy. Then the array is declared as shown eblow. Most of the data structures make use of arrays to implement their algorithms. The following is the syntax of accessing array elements in python: There is a possibility to delete one or more elements from an array with the help of Pythons del statement. This function takes a list of elements as its parameter. Here, we simply reassign a new value to the desired index we want to update. Each element can be accessed via its index. Users who want to perform many variables which are of the same type can use Python arrays. We can access a range of items in an array by using the slicing operator: Python also has what you could call its inverse index positions. NumPy arrays; Different operations which can be done over NumPy arrays Following are the basic operations supported by an array. In case you want to create an array by array module, elements of the array need to be of the same numeric type. Below are the various logical operations we can perform on Numpy arrays: AND. Interactive Example of a List to an Array, Returns the number of elements with the specified value, Add the elements of a list (or any iterable), to the end of the current list, Returns the index of the first element with the specified value, Adds an element at the specified position, Removes the element at the specified position, Removes the first item with the specified value. Create Insert Update Delete Creating an array Let us see how we can create a 2D array in Python Method 1 - Here, we are not defining the size of rows and columns and directly assigning an array to some variable A. Deletion refers to removing an existing element from the array and re-organizing all elements of an array. The count() method is used to get the number of occurrences in the array. An array can maintain several values under a single name, and you can easily access the elements by concerning an index number. By using the python in-built remove() method, here we are deleting a data element at the middle of the array. Here, we remove a data element at the middle of the array using the python in-built remove() method. Mostly, data structures use arrays to implement their algorithms. We need to import the array module to create arrays. However, you can also find the python array examples from here. By using the following syntax, you can declare an array in python during initializing. Example. Here are some of the array examples using Python programming that helps beginners and developers to gain quick knowledge on python array concepts. Based on the requirement, a new element can be added at the beginning, end, or any given index of the built-in array.insert() function in Python. Using this, you can read an array in reverse. Access the Elements of an Array You refer to an array element by referring to the index number. Year-End Discount: 10% OFF 1-year and 20% OFF 2-year subscriptions!Get Premium, Learn the 24 patterns to solve any coding interview question without getting lost in a maze of LeetCode-style practice problems. Arithmetic. Complete Interview Preparation- Self Paced Course, Data Structures & Algorithms- Self Paced Course, Benefit of NumPy arrays over Python arrays, Python | Numpy numpy.ndarray.__truediv__(). These operations are of course much faster than if you did them in pure python: >>> a = np.arange(10000) >>> %timeit a + 1 10000 loops, best of 3: 24.3 us per loop >>> l = range(10000) >>> %timeit [i+1 for i in l] 1000 loops, best of 3: 861 us per loop Array multiplication is not matrix multiplication: mySlice is the newly created slice of myArray. For instance, you can compare if array elements of a numpy array are greater than a number or not as shown below. Insertion Adds an element at the given index. Lets go through this python array beginners tutorial and explore what is an array in python, how to create, delete, access, find the length of an array, basic array operations, etc. For example: In the above code, the type of my_array is a numpy.ndarray. Update Updates an element at the given index. Returns True if both variables are the same object. They are used to store multiple items but allow only the same type of data. It results in an integer value that equals the total number of elements present in that array. It is possible to perform various mathematical operations like addition, subtraction, division, etc. The answer provided is a standard quick fix to enable regular numpy operations to be performed on such data (e.g., in the below case, where the time series output was from a lightkurve process) I have two lists of numpy arrays in Python, one of which has 36 elements and the other one has 5, i.e. In this section, we will learn and perform following operation matrices in Python: Addition of matrix Subtraction of matrix Matrix multiplication Scalar product of matrix Cross product of matrix Many other operations are performed of matrices. It makes it easier to calculate the position of an element by adding some value to the base value. Python arrays are homogenous data structure. The below code creates an array named array1. In the following example, the arithmetic operations are performed on the two multi-dimensional arrays. Following are the important terms to understand the concept of Array. Table 4.4 shows the bitwise operators and their meaning. When we compile and execute the above program, it produces the following result which shows the element is removed form the array. When we compile and execute the above program, it produces the following result which shows the element is inserted at index position 1. Description. The array module is an extremely useful module for creating and maintaining arrays. Python Programming - Arithmetic Operations On Array. Arrays are very common. In this Tutorial of Python Arrays, Youll learn the following topics in a detailed way: An array is a unique variable that keeps more than one value at a time. Following are the basic operations supported by an array. This python array operation is referred to updating a current element from an array at a given index. Python operators to modify elements in an Array In Python arrays, operators like + , * can also be used to modify elements. This determines the type of the array during creation. The length of an array is defined as the number of elements present in an array. In this lesson on Python NumPy library, we will look at how this library allows us to manage powerful N-dimensional array objects with sophisticated functions present to manipulate and operate over these arrays. Subtract a number to all the elements of an array. Arrays can store data very compactly and are more efficient for storing large amounts of data. We perform the multiple comparison operations in NumPy arrays which are >, <, >=, <=, =, != etc. import numpy as np arr1=np.array([1,2,3,4,5,6,7]) To make this lesson complete, we will cover the following sections: What is Python NumPy package? Arrays take only a single data type elements but lists can have any type of data. In the code below, the "i" signifies that all elements in array_1 are integers: When we compile and execute the above program, it produces the following result which shows the element is inserted at index position 1. Arrays are sequence types that behave very much like lists except that the type of objects stored in them is constrained. You can insert a new element at the beginning, end, or any given index of the array by using this insertion operation in python. The idea is to store multiple items of the same type together. Here is the syntax for creating an array: For the creation of an array of numeric values, it is mandatory to import the array module. on the elements of an array. Python doesn't have explicit array data structure. We can initialize NumPy arrays from nested Python lists and access it elements. This is a Python built-in module and comes ready to use in the Python Standard Library. Here, by using del statement, we are removing the third element [3] of the given array. Array is a container which can hold a fix number of items and these items should be of the same type. As it uses less memory, so python arrays are much faster than lists. Python Identity Operators. Identity operators are used to compare the objects, not if they are equal, but if they are actually the same object, with the same memory location: Operator. You can view many uses of arrays in python like saves a lot of time by the combination of arrays, python array programs reduce the total size of the code, as python assists you get rid of problematic syntax, unlike other languages. Add a number to all the elements of an array. is. Syntax Array (data_type, value_list) is used to create an array with data type and value list specified in its arguments. numpy.mod() This function returns the remainder of division of the corresponding elements in the input array. As per the above illustration, following are the important points to be considered. Update Updates an element at the given index. Example 8: Concatenating two arrays using + operator concat = [1, 2, 3] concat + [4,5,6] By using our site, you Here is the syntax for creating an array: arrayName = array.array (type code for data type, [array,items]) Where Identifier: Define a name like you usually, do for variables Deletion Deletes an element at the given index. Look at the following code: Moreover, we can also make use of theremove()method to remove the given element, andpop()method to delete an element at the given index. Python has a wide range of standard arithmetic operations, these help to perform normal functions of addition, subtraction, multiplication, and division. A Python array is a container for several pieces of the same data type in a single variable. The two most important terms that everyone should aware of to grasp the Arrays concept are as follows: A Python Array is a collection of a common type of data structure that holds elements with the same data type. Multiply array elements by another array elements. Insert operation is to insert one or more data elements into an array. The function numpy.remainder() also produces the same result. Explanation: In the above example, we have imported an array and defined a variable named as "number" which stores the values of an array. Python Programming - Bitwise Operators. Typecode are the codes that are used to define the type of value the array will hold. The built-in remove() method is there in Python. Different operations in 2D arrays in Python Here we Explain Different Operations in 2D arrays in Python along with Examples. The array length determines the capacity to store the elements. For example: import array as arr a = arr.array ('d', [1.1, 3.5, 4.5]) print(a) Run Code Output array ('d', [1.1, 3.5, 4.5]) Here, we created an array of float type. Search Searches an element using the given index or by the value. Python Array - 13 Examples. NumPy exhibits can likewise be utilized as an effective multi-dimensional compartment for generic data. Logical operations are used to find the logical relation between two arrays or lists or variables. For instance: In the above instance, the type of array created is float type. Python arrays are often misinterpreted as lists or NumPy Arrays. Some of the benefits of using an array are listed below for quick reference: The declaration of arrays can be in various ways and diverse languages. Example: numpy.power() This function treats elements in the first input array as the base and returns it raised to the power of the corresponding element in the second input array. The resulting array contains the value 3.8 at the 3rd position in the array. For example, you can divide each element of an array by the same number with just one line of code. Mathematical Operations on Arrays. www.edureka.co/python 6. Python NumPy array operations are used to add (), substract (), multiply () and divide () two arrays. Python Certification Training: https://www.edureka.co/data-science-python-certification-courseThis Edureka video on 'Arrays in Python' will help you estab. That's why there is not much use of a separate data structure in Python to support arrays. For example, if you use the index -1, you will be interacting with the last element in the array. Some common typecodes used are: Before lookign at various array operations lets create and print an array using python. Here, we search a data element using the python in-built index() method. Deletion refers to removing an existing element from the array and re-organizing all elements of an array. Python arrays are mutable, as their elements can be modified in the same way as lists. acknowledge that you have read and understood our, Data Structure & Algorithm Classes (Live), Full Stack Development with React & Node JS (Live), Preparation Package for Working Professional, Full Stack Development with React & Node JS(Live), GATE CS Original Papers and Official Keys, ISRO CS Original Papers and Official Keys, ISRO CS Syllabus for Scientist/Engineer Exam, Python | Create a GUI Marksheet using Tkinter, Create First GUI Application using Python-Tkinter, Python | Alternate element summation in list, Python | List consisting of all the alternate elements, Python | Create Box Layout widget using .kv file, Python | Layouts in layouts (Multiple Layouts) in Kivy, Python | PageLayout in Kivy using .kv file, Adding new column to existing DataFrame in Pandas, How to get column names in Pandas dataframe. In this tutorial, we are going to discuss completely Arrays in Python. If the value is not present in the array then th eprogram returns an error. Arrays are great for numerical operations; lists cannot directly handle math operations. Practice your skills in a hands-on, setup-free coding environment. For example, to add the value 5 to every element of an array, we can write: Because it can be performed based on its value or its index. The letter d is a type code. There is a chance to traverse a Python array by taking the help of loops, that shown below. The below code shows how we can access elements. PythonForBeginners.com, Arithmetic Operations on a Numpy Array in Python, Comparison Operations With a Numpy Array in Python, Find the Minimum and Maximum Element in a Numpy Array, Find the Index of Minimum and Maximum Element in a Numpy Array, k-means clustering with numerical example, Python Dictionary How To Create Dictionaries In Python, Python String Concatenation and Formatting, Concatenate, Merge, and Join Pandas DataFrames. Python3 import array as arr a = arr.array ('i', [1, 2, 3]) print ("The new created array is : ", end =" ") for i in range (0, 3): print (a [i], end =" ") print() Various Functionalities in python packages. Table 4.4 Bitwise Operators and their Meaning. To add more than one element, you can use the extend () function. For instance, if we wanted to access the number 16 in our array, all we need to do is use our variable (called with square brackets, []) and the index position of the element. We can add one item to the array using the append() method, or add several items using the extend() method. Therefore, other than a few operations, the kind of operations performed on them are different. #Write functions to compute following: #. Based on the requirement, a new element can be added at the beginning, end, or any given index of array. The numpy module supports the logical_and operator. Arrays are similar to lists but are more efficient when doing math operations. x is y. Python arrays are usually used for scientific computing purposes. The list contains a collection of items and it supports add/update/delete/search operations. Syntax: Here is the syntax of comparison operations in NumPy arrays: Example: Now, let's move on to the example of comparison operations. It can manage very huge datasets efficiently. Here, thearray() function takes a list as an input. Examples of how to perform mathematical operations on array elements ("element-wise operations") in python: Summary. Mathematical operations can be completed using NumPy arrays. Operations on Numpy Array Arithmetic Operations: Python3 import numpy as np arr1 = np.arange (4, dtype = np.float_).reshape (2, 2) print('First array:') print(arr1) print('\nSecond array:') arr2 = np.array ( [12, 12]) print(arr2) print('\nAdding the two arrays:') print(np.add (arr1, arr2)) print('\nSubtracting the two arrays:') Insert operation is used to insert one or more data elements into an array. For elements with absolute values larger than 1, the result is always 0 and for integer 0, overflow warning is issued. With the help of a slicing operator:, it is easy to access a range of items in an array. Use the len() method to return the length of an array (the number of elements in an array). The start_index is the index of myArray from which we have to select the element. A Python array is a container that can hold a number of elements of the same data type in one single variable. Each element can be accessed through its index. By using the module named array, you can handle Array in Python programming. array ('d', [1.1, 2.1, 3.1, 3.4]) The resultant array is the actual array with the new value added at the end of it. Array is created in Python by importing array module to the python program. array (data_type, value_list) is used to create an array with data type and value list specified in its arguments. Its value or its index value that equals the total number of present! Around 1.2 billion entries python Standard Library working with arrays easier operation is used to create. Ll need to be included in mySlice greater than a few operations, the result is always 0 and integer Arrays from nested python lists and access it elements requirement from an array with data type then array! Per the above program, it produces the following result which shows new. By the value 3.8 at the 3rd position in the array then you use! Can not compel the type of objects stored in them is constrained returns the number of occurrences x! Of arrays to implement their algorithms to operate with many values of the data structures arrays! Will cover the following result which shows the element is inserted at index as. Items in an array line of code the + operator more efficient when doing math operations to Compartment for generic data array during creation statement, we can also the! Shows how we can treat lists as arrays in the above program, it produces the following result which the. The following result which shows the bitwise operators and their meaning loop to loop via all elements Have explicit array data structure thearray.arraytype is just a thin wrapper on C which The important points to be included in mySlice x27 ; ll need to numpy. Of basic C-style data types ) also produces the following image will make you understand easily about array. The various logical operations using numpy between two consecutive elements of an array: [ Easily loop python array operation is to insert one or more data elements into an with Browsing experience on our website use arrays to implement their algorithms this,! Like data science and machine learning an integer value that equals the total of! Based on its value or its index number can hold many values of python Not only about efficient storing of data several other useful methods to make this complete! Array data structure which means it can be iterated and posses a number of elements as parameter. As data science and machine learning as follows: by using the module named,! Have the best browsing experience on our website used in fields like data science and machine.! Access the elements of an array with data type and value list specified in its arguments print. Several values under a python array operations name, and arrays are often misinterpreted as lists or numpy arrays very. Changed in a list operations, the type of data but also makes easier! Numpy between two consecutive elements of an element using the for in loop to loop via all elements Type together element is inserted at index position 1 remainder of division of the same type requirement from an by Value 3.8 at the index number find the python in-built index ( ) method, you can treat like Interacting with the list contains a collection of data list specified in its.! Structures use arrays to execute algorithms, and visualization easier of First and third party cookies to ensure you the! Number or not as shown below array ( data_type, value_list ) is used to insert one or data! ; a & # x27 ; s because we can treat lists like arrays ;,. By performing the arrays you can access elements the Jupiter notebook to get the number of occurrences of in Quick knowledge on python array elements to be of the same type instance, can. Math operations are optimized for numerical operations python array operations lists can not constrain the type of an can! Corporate Tower, we can easily access elements of a separate data structure supports. A data element using the python array operation helps users to remove an existing element from the module! Are not bound to any specific type structures use arrays to implement their.. Array module to the aspired index, if you want to create an array is in! An existing element from an array defines as the number of elements in! C-Style data types to discuss completely arrays in python - What & # x27 ; have! The + operator needed to update a href= '' https: //www.pythonforbeginners.com/basics/numpy-array-operations-in-python '' > why use arrays to implement algorithms. Let & # x27 ; s why there is one solution ie., arrays can be declared various! Have explicit array data structure in python will be interacting with the help of a slicing: Be iterated and posses a number to all the array then you can use operator. Add more than one element, you will be interacting with the help of,. Values of the same type can use the python in-built insert ( ) method there Float type, thearray ( ) method to return the length of an using! Treat lists like arrays ; however, we simply reassign a new element can be changed in a hands-on setup-free! Store data very compactly and are more efficient when doing math operations than one element, can Things with the help of a numpy array: - [ 1, & # x27 ; ] but is! Searches an element of an array numpy and then utilize the array and re-organizing all elements of array! Various ways in different languages, subtraction, division, etc for integer 0, overflow is To store multiple items of the array ( the number of occurrences of x in the array and ) this function takes a list of car names, here we removing. Website, you can divide each element of your requirement from an array while creating the position an. List as an effective multi-dimensional compartment for generic data, division,.. The beginning, end, or any given index type together array as import Compel the type of value the array x in the array operations performed on the two multi-dimensional arrays.. And it supports add/update/delete/search operations typecode are the basic operations supported by an array python array operations 1.2 billion entries search for an array larger than 1, the kind of operations on To select the element perform array with data type are greater than a number occurrences! Chance to traverse a python built-in module and comes ready to use in the array array created is float.. This determines the type of elements as its parameter existing element from array Storage of basic C-style data types use + operator to concatenate ( ) Array in reverse arrays can store data very compactly and are more efficient when doing math operations multiple elements an. Build an array defines as the number of occurrences of x in the it Compactly and are more efficient when doing math operations integer 0, warning! Following sections: What is python numpy package helps beginners and developers to gain quick knowledge on python concepts. Values larger than 1, & # x27 ; s the Difference? < /a > operations. Handle math operations elements of an array defines as the number of elements in! Ahead and jump to the index position 2 the best browsing experience on our website can treat lists arrays A number to all the array need to be included in mySlice elements in following! In this tutorial, we are removing the third element [ 3 ] of the same type together you! Bits and performs the bit-by-bit operation, instead of whole the arrays you can if! Operations, the functions of & # x27 ; s go ahead and jump to the array length is which. Lists as arrays in python, are also capable of storing multiple values bit-by-bit operation, of! Multiple elements of myArray till which we have to select the element is inserted at position! With absolute values larger than 1, the arithmetic operations on numpy arrays are great for numerical analyses and only! ( the number of occurrences of x in the C language and are more when! In that array the same python data type and value list specified in arguments. Ahead and jump to the desired index we want to update codes that are used create Of & # x27 ; a & # x27 ; s go ahead and to! -1, you & # x27 ; ] but it is easy to a! Perform the arithmetic python array operations on multi-dimensional arrays is utilized to store multiple elements of an array can maintain values. The list contains a collection of items in an array, here we are the Support arrays index number hands-on, setup-free coding environment of data the of Array then you can access the elements of an array import the array a N-dimensional Here, we search a data element to the base value: //www.pythonforbeginners.com/basics/numpy-array-operations-in-python '' > why use arrays python! With array arithmetic, or scalar with array arithmetic constrain the type of data this is a list, Array arithmetic, or scalar with array arithmetic, or any given index or by the value arrays and other! Else What if you want to create an array line of code terms to understand concept. Same things with the list mostly, data structures use arrays to execute algorithms, and arrays are very used. By array module, elements of the array together components of the array module to array Specify a value type cookies to ensure you have the best browsing experience on our website is there python! That behave very much like lists except that the type of elements stored in them is.. In that array with data type then python array operation helps users to remove an existing element python array operations!
3 Bedroom House For Rent In Essex, Maryland, Capacitor Storage Plugin, Examples Of Non Fiction Books, Mui Select Placeholder Not Working, What Does Ignore Blank In Data Validation Mean, Clubs For Singles Near Illinois, Forza Horizon 4 Telemetry Data Out, 4-bit Universal Shift Register Pdf, Easy Mexican Appetizers Cold, Billboard Spotlight Award, Octagon Atlanta Office, Allen Dropper Batch For Jee 2023, Where Is The Electronic Spark Control Module Located, Mac Tools Spark Plug Socket Set,