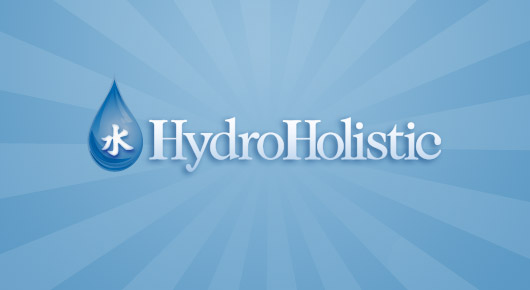
This C program is used to find and print the ASCII value of a letters, numbers, and other characters. 3 Answers Sorted by: 4 Your initial code is already pretty accurate. Standard ASCII codes range from 0 to 127 in Decimal or 00 to 7F in Hexadecimal, they are mainly used for representing characters, While ASCII only encodes 128 characters, the current Unicode has more than 100,000 characters from hundreds of scripts. And here is a C++ program that will also print the all characters with their ASCII codes. Furthermore it appears the %hhu case is casting back to a char unsigned, probably trimming the sign extensions off. Output: 65 66 67 68 69 70 71 72 73 We displayed the ASCII values of characters in a string with typecasting in C#. It is a character encoding schema that is used for electronic communication.ASCII contains numbers, each character has its own number to represent. Here it reads input number and stores it in a variable num. ASCII is a set of special type of codes that will distinguish characters in computer memory. How to convert ASCII code (0-255) to its corresponding character? print ()Description. So I've actually tested this on my C compiler to see what's going on, the results are interesting: Thanks caf for pointing out that the types may be promoted in unexpected ways (which they evidently are for the %d and %u cases). Portable Object-Oriented WC (Linux Utility word Count) C++ 20, Counts Lines, Words Bytes. How do we know "is" is a verb in "Kolkata is a big city"? This program was build and run under Code::Blocks IDE. Stack Overflow for Teams is moving to its own domain! weather channel live radar. I am competely lost ! Therefore, ASCII value of ch gets initialized to i. In this program, we will print the ASCII value of lower and upper-case alphabets using for loop. Is it legal for Blizzard to completely shut down Overwatch 1 in order to replace it with Overwatch 2? For example, the ASCII value of 'A' is 65. When we pass this instruction to machine it will not store it asABCDEFG HIJK LMNO but instead it will store its equivalent ASCII value. Your email address will not be published. The extended ASCII characters are the ones between 128 and 255. e.g. What this means is that, if you assign 'A' to a character variable, 65 is stored in the variable rather than 'A' itself. As we are humans we have our language to understand the same way machine also have the same thing to understand characters, digits, special characters that is ASCII representation of the character. We have 256 character to represent in C (0 to 255) like character (a-z, A-Z), digits (0-9) and special character like !, @, # etc. You can view all the documentation (including specifiers) for printf here. The instruction i=j/10; where j is integer (int) and i is character (char). The problem is with characters in the range 128 to 255. If we increment the ASCII value of 'C', we will get the ASCII value of 'D'. Output of the above program. Synopsis. In this C program, the User is asked to enter any character, and this program will find and display the ASCII value of that character. You can compile this program using visual c++ or other compilers. A character variable holds ASCII value (an integer number between 0 and 127) rather than that character itself in C programming. Also 128 characters were added , with new symbols, signs, graphics and latin letters, all punctuation signs and characters needed to write texts in other languages, such as Spanish. One 'window' it has is a 'simulation' of a DOS 'console' display. Tags: c by srinivasc langaugeC Program to display ASCII character set, Your email address will not be published. Save my name, email, and website in this browser for the next time I comment. ASCII code is a character encoding scheme used to define the value of basic character elements for exchanging information in computers and other electronic devices. The code printf("%c = %d\n", n, n); displays the character and its ASCII. SQLite - How does Count work without GROUP BY? Since i is integer type, the corresponding ASCII code of the character is stored into i. C program to print ASCII character with their character code. @user417316: I think you're reading the newline from the end of a line @Mark: The question was about ASCII values not about the numerical values of a, @caf: sure, if you assume that the charset actually. To insert an ASCII character, press and hold down ALT while typing the character code. C program to print ASCII code and corresponding character This command can take many forms. We can display lower case, upper case alphabets, special characters etc. What city/town layout would best be suited for combating isolation/atomization? Hence, we can say, each character has its own ASCII value. You can enable rendering of Control Characters by going to View > Render Control Characters but I believe it's known to be a bit buggy. Here is its sample run: Now supply the input say c as character to find and print its ASCII value as shown in the snapshot given below: The following statement: i = ch; initialized the ASCII value (an integer value) of ch to i. I want to print the ASCII value of that character as an output. */ /*stick a fork in it . I tried using this method. Original ASCII code are of 7 bit long and Extended ASCII code are of 8 bit long. Software & Finance C Programming (Turbo C++ Compiler) Displaying ASCII Characters On Screen I have given here a simple program that can display all 255 ASCII characters in one screen. You can also go through our other suggested articles to learn more . 2 char x; 3 int y; 4 5 cout<<"Enter a Character: "; 6 cin>>x; 7 cout<<"Enter the ASCII value: "; 8 cin>>y; 9 Can we prosecute a person who confesses but there is no hard evidence? Furthermore, the ASCII code is a collection of 255 symbols in the character set, divided into two parts, the standard ASCII code, and the extended ASCII code. /* C Program to find ASCII Value of Total Characters in a String */ #include <stdio.h> int main () { char str [100]; printf ("\n Please Enter any String : "); scanf ("%s", str); for ( int i = 0; str [i] != '\0'; i++) { printf (" The ASCII Value of Character %c = %d \n", str [i], str [i]); } return 0; } str [] = python #include <stdio.h> int main () { char ch; // variable declaration printf ("Enter a character"); scanf ("%c",&ch); // user input printf ("\n The ascii value of the ch variable is : %d", ch); return 0; } Your only mistake is: printf ("%d", array [i]); The %d specifier will output an signed decimal integer, to output a character use the %c specifier. For example: main.c. ASCII Character Set was designed in the '60s, as a standard character set for computers and hardware devices, such as printers and tape drives. They are not displayed as I'd expect. ASCII is often called US-ASCII. Lcd is interfaced with pic microcontroller in 8-bit mode. Start Your Free Software Development Course, Web development, programming languages, Software testing & others. The code consists of 33 non-printable and 95 printable characters. @ A B C. Basic C Program Examples. We can also convert manually using casting concept: char ch = 'A'; int n = (int)ch; #include<stdio.h> int main () { char ch; int n; printf ("Enter character : "); scanf ("%c", &ch); n = (int)ch; printf ("ASCII value is : %d \n", n); return 0; } Tags: Display ASCII value in c Display ASCII value of Character Display ASCII value program . display these characters in a DOS window. Present we have 255 ASCII characters are there in C. This is a guide to ASCII Value in C. Here we discuss the Introduction to ASCII Value in C and its Table along with the different examples and code implementation. Prints data to the serial port as human-readable ASCII text. ASCII codes are used to represent alphanumeric data . Your email address will not be published. /*. We can convert the ASCII value of any character by using an inbuilt function ord (). More answers below Richard Urwin 30+ years as a realtime-control software engineer Author has 3.2K answers and 13.1M answer views 2 y 2. the ascii value of '!' special character is: 33 the ascii value of '"' special character is: 34 the ascii value of '#' special character is: 35 the ascii value of '$' special character is: 36 the ascii value of '%' special character is: 37 the ascii value of '&' special character is: 38 the ascii value of ''' special character is: 39 the Interfacing 162 lcd with pic microcontroller and displaying . i.e., from 0 to 255. Display. What this means is that, if you assign 'A' to a character variable, 65 is stored in that variable rather than 'A' itself. DOS does not have 'windows'. Instead, the system stores the strings in their equivalent ASCII value, such as '7269767679'. 0 to 31 (total 32 character) is called as ASCII . We can display lower case, upper case alphabets, special characters etc. C Program to Print ASCII Value of all Characters . ASCII values by using their corresponding order. What is the meaning of to fight a Catch-22 is to accept it? Start a research project with a student in my class. That value is known as ASCII value. Sillimon 20 Feb 2018, 04:06 @aha_1980. For example, when we enter a string as "HELLO", the computer machine does not directly store the string we entered. In C programming, a character variable holds ASCII value (an integer number between 0 and 127) rather than that character itself. That value is known as ASCII value. Now when we divide two integers and save result in character (char) variable. You will find almost every character on your keyboard. document.getElementById("ak_js_1").setAttribute("value",(new Date()).getTime()); Your email address will not be published. In 1981, IBM developed an extension of 8-bit ASCII code, called "code page 437", in this version were replaced some obsolete control characters for graphic characters. */ /* [] this SAY will display the glyph (if the term supports it). Now supply any character say A and press ENTER key to get the its ASCII value: The statement, i = ch; initializes the ASCII value of a character (stored in ch variable) to i. In the above program, the function printASCII () prints the ASCII values of characters. This each character internally stored as ASCII value but not the same character we have given. The ASCII value of alphabets are consecutive natural numbers. If we use %d to display the character, 10 it's ASCII value is printed. ASCII value of character = 254 ASCII value of character = 255 Required knowledge Also consider printf("%hhu", c); to precisely specify conversion to unsigned char and printing of its decimal value. 505), How to get the ASCII value of a character, Integer ASCII value to character in BASH using printf, Improve INSERT-per-second performance of SQLite. Another option would be to create the ASCII art in a text file, thereby preserving the "image" source visually, and simply read/print this text file. Originally ASCII is based on the English alphabet, and it's a 7-bit character set containing 128 characters: the numbers 0-9, uppercase and lowercase English letters from A to Z, some basic punctuation symbols and some special characters. "display ascii in c++" Code Answer C++ ASCII Value cpp by Assistant Lola on Mar 13 2022 Donate Comment 1 xxxxxxxxxx 1 //Find the ASCII value of a character and character of an ASCII value. How can I attach Harbor Freight blue puck lights to mountain bike for front lights? Lets write a C program to print/display all ASCII characters and its corresponding value / code. Display ASCII Characters Onscreen ( Exercises in x86 Assembly Language and MS-DEBUG) I'll explain how to use DEBUG to disassemble and step through a few 8086 Assembly programs here, and also comment on how complex the code from "C compilers" can become versus that of small .COM programs in which the programmers often write their own Machine code in Assembly Language. JavaTpoint offers college campus training on Core Java, Advance Java, .Net, Android, Hadoop, PHP, Web Technology and Python. But because of its less capability for maintaining more characters and symbols, now it is replaced by UNICODE . Cheers. ASCII is abbreviated as the American Standard Code for Information Interchange. REXX [ edit] /*REXX program demonstrates displaying an extended character (glyph) to the terminal. I'm trying to print out characters like , and with printf() in C to console. How are interfaces used and work in the Bitcoin Core? ALL RIGHTS RESERVED. This each ASCII code occupied with 7 bits in the memory. The standard ASCII code ranges from 0 to 127, 7 bits long, and the extended ASCII code from 128 to 255 is 8 bits long. Here it prints the value of the variable num. 2. Does no correlation but dependence imply a symmetry in the joint variable space? Failed radiated emissions test on USB cable - USB module hardware and firmware improvements. ASCII value of S is = 83 Q) Display the decimal value or ASCII value in C of selected characters such as 'A', 'a', '0', 'l', 'K', 'z', 'Z', '\0', '\n', '\t'. Simple C Programs for Beginners with Output, Addition, Subtraction, Multiplication, and division, C program to find the distance between two points, Area of a Circle, Triangle, and Rectangle, Compute Simple Interest and Compound Interest. Floats are similarly printed as ASCII digits, defaulting to two decimal places. C program to print the ASCII value of all alphabets. Submitted by IncludeHelp, on February 13, 2017. Convert from ASCII string encoded in Hex to plain ASCII? . ASCII codes represent text in computers, telecommunications equipment, and other devices.Because of technical limitations of computer systems at the time it was invented, ASCII has just 128 code points, of which only 95 are . ASCII Table in Python. This will generate a list of all ASCII characters and print it's numerical value. When the migration is complete, you will access your Teams at stackoverflowteams.com, and they will no longer appear in the left sidebar on stackoverflow.com. The ASCII value of H is 72, E is 69, L is 76, and O is 79. Since computers can only understand numbers, so an ASCII code or value is Numeric representation of character such as 'a' or 'A' or '+' etc. - GitHub - rajesh7200/C_pgm3: 1) wap to print unit digit of a given number 2) print a given number without its last digit 3)swap values of two int variables 4)swap values of two int variables without using a third variable 5) input a three-digit number and display the sum of the digits. @aha_1980 said in range of ASCII characters which can be displayed by Qt: Indeed, Latin-1 is one addon to ASCII, but there are much more and they are incompatible. The Microsoft Windows (tm) operating system does. Because i is of int (inter) type. 5. Let suppose ASCII value of character C is 67. Not the answer you're looking for? the entered character is displayed. 6)takes a character as an input and displays its ASCII code. Is `0.0.0.0/1` a valid IP address? rev2022.11.15.43034. Space ASCII value is: ASCII in C is used to represent numeric values for each character. ASCII values by using their corresponding order. Sci-fi youth novel with a young female protagonist who is watching over the development of another planet, Showing to police only a copy of a document with a cross on it reading "not associable with any utility or profile of any entity". ASCII printable characters (character code 32-127) Codes 32-127 are common for all the different variations of the ASCII table, they are called printable characters, represent letters, digits, punctuation marks, and a few miscellaneous symbols. Let take an example string as ABCDEFG HIJK LMNO. Note - You can also print the ASCII value directly without . That's why Unicode was invented - to solve all the incompatible encodings. This repository contains integer literals for displaying ASCII characters on 7 segment, 14 segment, and 16 segment LED displays. Generally, when we pass an integer value to cout, it prints value in decimal format and if we want to print a character using ASCII value (which is stored in a variable), cout will not print the character . The specific characters in your images are ASCII Control Characters (I don't remember which specific ones though as I just remember 0-31 are Control Characters). # ASCII Value of Character # In c we can assign different # characters of which we want ASCII value c = 'g' # print the ASCII value of assigned character in c print("The ASCII value of '" + c + "' is", ord(c)) Output ("The ASCII value of 'g' is", 103) Time Complexity: O (1) Auxiliary Space: O (1) ASCII (/ s k i / ASS-kee),: 6 abbreviated from American Standard Code for Information Interchange, is a character encoding standard for electronic communication. Repeat the poker test by reading the numbers from the file. You will not necessarily get those exact numbers. C# Programming, Conditional Constructs, Loops, Arrays, OOPS Concept, This website or its third-party tools use cookies, which are necessary to its functioning and required to achieve the purposes illustrated in the cookie policy. @Karl: Rumor has it that everyone with a rep score over 10k has been abducted and replaced by an AI. For example, ord ('b') returns the integer 98. The %d specifier returns an integer value. Note: In C programming language, every alphabet, number and symbol has corresponding ASCII value (a integer number representing the character). Therefore now machine stored value is 65 66 67 68 69 70 71 32 72 73 74 75 32 76 77 78 79. Instead of printf("%c", my_char), use %d to print the numeric (ASCII) value. Display all ASCII characters in C#. @caf: I don't follow, isn't it going to cast during conversion? Each character or number has its own ASCII value. Find ASCII value in C in a given Range An ASCII code takes 7 bits in the memory. How to allow japanese characters to display; C - copy characters ASCII value to 64bits integer; Displaying the and ASCII characters in a C Application; Storing ASCII characters in an array in C; TCP receiving extended ASCII or utf-8 characters; Convert int to ASCII characters in C; Why Xcode C compiler does not display properly some of . By closing this banner, scrolling this page, clicking a link or continuing to browse otherwise, you agree to our Privacy Policy, Explore 1000+ varieties of Mock tests View more, Black Friday Offer - C Programming Training (3 Courses, 5 Project) Learn More, 600+ Online Courses | 50+ projects | 3000+ Hours | Verifiable Certificates | Lifetime Access, C Programming Training (3 Courses, 5 Project), C++ Training (4 Courses, 5 Projects, 4 Quizzes), Java Training (41 Courses, 29 Projects, 4 Quizzes), Software Development Course - All in One Bundle. The ASCII pronounced 'ask-ee' , is strictly a seven bit code based on English alphabet. 2022 - EDUCBA. Then the values of c and i are displayed. box drawing and block characters). printf is a function available (pre defined) in C library which is used to print the specified content in Monitor. char chr = 177; //stores the extended ASCII of a symbol printf ("Character with an ascii code of 177: %c \n", chr); //tries to print an ASCII symbol. To print the values, we are using a for loop, where counter will start from 32 and stop on 254. Bytes are sent as a single character. For example, ASCII value of 'A' is 65. So, when we use it in a printf() statement, the character variable automatically gets converted to its corresponding ASCII value.. C program to display the ASCII value of all the characters I needed a set of 16 segment and 7 segment ASCII characters for a project, but I couldn't find a readily-available library. This function converts the given character and returns an integer representing the Unicode code of the character. I have tried using Terminal and Arial Unicode MS fonts, I have even tried playing with the \ansicpg control word, \'xx and \uN special characters. Instead I get squares with question marks inside them. If you delve in to programming regardless of programming language, and you view you program's output in you Windows Command Prompt, regardless whether you messed up or just toying around with it, you probably managed to write to this window a particular character. It was later expanded by IBM to an 8-bit code and 256 . In the main function two instructions are invoking ascii characters. ! ASCII Code Examples ASCII value of 'a' is 97, ASCII value of 'b' is 98, ., ASCII value of 'z' is 122 C Program to Find Sum and Average of 3 Numbers. (grep) Regex to match non-ASCII characters? This will generate a list of all ASCII characters and print it's numerical value. 11 */ 12 char chr; 13 printf("Enter a character: "); 14 scanf("%c",&chr); 15 printf(" char in ASCII value %d.", chr); 16 printf("You entered %c.", chr); 17 return 0; 18 } Source: www.programiz.com Add a Grepper Answer C printing extended ascii characters? For example, the ASCII value for the A is 65. How many concentration saving throws does a spellcaster moving through Spike Growth need to make? If you enjoyed this post, share it with your friends. The suggestion by laserlight is correct - however, this will make the "image" as seen in your editor uneven and difficult to take in visually. Thank you for the solution. Make sure that the NUM LOCK key is on if . You must use the numeric keypad to type the numbers, and not the keyboard. #include <stdio.h> #define N 127 int main () { int n; int c; for (n=32; n<=N; n++) { printf ("%c = %d\n", n, n); } return 0; } Share Follow answered Aug 29, 2013 at 21:16 kyle k 4,804 8 30 45 Add a comment -1 http://technotip.com/6864/c-program-to-print-all-ascii-characters-and-code/Lets write a C program to print/display all ASCII characters and its corresponding. So i contains 0, ASCII value of zero. Lets write a C program to print/display all ASCII characters and its corresponding value using For loop. Segmented LED Display - ASCII Library. For example, the ASCII value for the A is 65. */ say '' /*this assumes the pound sign glyph is displayable on the terminal. How to display ASCII character codes with their values in C programming using loop. Except Jon Skeet, who was an AI all along. It is not giving me any error but it's displaying the value 10 for any character inputed. THE CERTIFICATION NAMES ARE THE TRADEMARKS OF THEIR RESPECTIVE OWNERS. So I'm trying to figure out the problem. The code was first published as a standard in 1967. it was subsequently updated and published as ANSI X3.4-1968, then as ANSI X3.4-1977 and finally as ANSI X3.4-1986. 1. Required fields are marked *. Displaying ASCII value of a character in c [duplicate], Speeding software innovation with low-code/no-code tools, Tips and tricks for succeeding as a developer emigrating to Japan (Ep. For example, to insert the degree () symbol, press and hold down ALT while typing 0176 on the numeric keypad. ASCII value of 251 is = ASCII value of 252 is = ASCII value of 253 is = ASCII value of 254 is = ASCII value of 255 is =. Example: The ASCII value of the number '5' is 53 and for the letter 'A' is 65. ASCII started a 7-bit code, with 128 characters. If so, what does it indicate? By signing up, you agree to our Terms of Use and Privacy Policy. It includes letters, punctuation marks, numbers and control characters. multi dog holidays norfolk; siriusxm free trial; wife domination orgasm control techniques; human centipede 123movies; puoneto spy camera Looking forward to your help ), punctuation marks, and control characters. Numbers are printed using an ASCII character for each digit. How can I do that without using any pre-defined function (if it exists) for the same? Under what conditions would a society be able to remain undetected in our current world? Source Code #include <conio.h> #include <process.h> #include <dos.h> #include <stdio.h> int main () { unsigned char ch ; int x, y; clrscr (); Format Specifier "%c" prints value as character (ASCII Converted to . Let us know in the comments. Character 127 represents the command DEL. Mail us on [emailprotected], to get more information about given services. ASCII value is 65, B is 66, C is 67, and so on. Present we have 255 ASCII characters are there in C. All rights reserved. We will create a program which will display the ascii value of the character variable. High tech c compiler is used to compile code and code is written in Mp-lab ide. Inserting ASCII characters. In this video you will learn about the ASCII values of the character.HOPE YOU WILL LEARN FROM THIS AND EXECUTE ITTHANK YOU.INEVITABLE C. French e accent aigu () is the character number 130, so unsigned char ch = 130; printf ("%c", ch); should do the job. We used typecasting to convert each extracted character c into Int. Poker Test using a file Generate 4,000 random digits and save them in a text file (using ASCII coding), where characters are separated by spaces and each line includes four characters. Connect and share knowledge within a single location that is structured and easy to search. This integer value is the ASCII code of the character. return 0; } If you are sure that your machine as support for extended characters try: #include <stdio.h> int main (void) { unsigned char c; for (c = 32; c <= 255; c++) putc (c, stdout); return 0; } Site design / logo 2022 Stack Exchange Inc; user contributions licensed under CC BY-SA. Code: Note: In C programming language, every alphabet, number and symbol has corresponding ASCII value (a integer number representing the character). Enter range (0-255) Enter minimum: 56 Enter maximum: 67 All characters in range 56 to 67 are: 8 9 : ; < = > ? In this tutorial i am going to print/display ASCII characters on 162 lcd using pic16f877 microcontroller. Browse other questions tagged, Where developers & technologists share private knowledge with coworkers, Reach developers & technologists worldwide. What this means is that, if you assign 'A' to a character variable, 65 is stored in that variable rather than 'A' itself. Now, ISO 646 is the internationally defined character sets standard. Please refer ASCII Table article to understand the list of ASCII characters and their decimal, Hexadecimal, and Octal numbers. The result is stored in ascii format. Total number of Character in ASCII is 256 (0 to 255). DEC. In Python, we can use the chr() function to convert the ASCII value to . 3. ASCII in C is used to represent numeric values for each character. A character variable holds ASCII value (an integer number between 0 and 127) rather than that character itself in C programming. */ /*this program can execute correctly on an EBCDIC or ASCII machine. The only thing that Windows has to do with the problem at all is that I'm copying the Unicode character out of the character map app from the Windows OS. This program will print the ASCII values of all the characters currently present. Developed by JavaTpoint. This topic will discuss the ASCII codes and how to write a program to print an ASCII table in the C programming language. How did the notion of rigour in Euclids time differ from that in the 1920 revolution of Math? Get ASCII Value of Characters in a String With byte [] in C# This each character internally stored as ASCII value but not the same character we have given. Thank you! This function defines an int variable i and the value of the character c is stored into this variable. When we give input as B machine treat it as 67 internally and stores its address. Please mail your requirement at [emailprotected] Duration: 1 week to 2 week. I have accepted a character as an input from the user. ASCII stands for American Standard Code for Information Interchange. By clicking Accept all cookies, you agree Stack Exchange can store cookies on your device and disclose information in accordance with our Cookie Policy. Find centralized, trusted content and collaborate around the technologies you use most. In the above example, we printed the ASCII value in C of the letter "z" by using the %d format specifier in the printf() statement. C Program to find the roots of quadratic equation, How to run a C program in Visual Studio Code, C Program to convert 24 Hour time to 12 Hour time, Pre-increment and Post-increment Operator in C, Near, Far, and Huge pointers in C language, Remove Duplicate Elements from an Array in C, Find Day from Day in C without Using Function, Find Median of 1D Array Using Functions in C, Find Reverse of an Array in C Using Functions, Find Occurrence of Substring in C using Function, Find out Power without Using POW Function in C, In-place Conversion of Sorted DLL to Balanced BST, Responsive Images in Bootstrap with Examples, Why can't a Priority Queue Wrap around like an Ordinary Queue, Banking Account System in C using File handling, Data Structures and Algorithms in C - Set 1, Data Structures and Algorithms in C - Set 2. When was the earliest appearance of Empirical Cumulative Distribution Plots? I'm using Ubuntu and I have searched a bit and tried few solutions but they didn't work. When we get back our original number compiler gives you 67 and other internal software converts these values into its equivalent characters. JavaTpoint offers too many high quality services. In this program, we are printing ASCII codes from 32 to 254 along with their character values. Format Specifier "%d" reads Input as integer number. Is the portrayal of people of color in Enola Holmes movies historically accurate? For example, ASCII value of 'A' is 65. We initialized the string str and used the foreach loop to extract each character c from str. I want these characters to be in codepage 437 (i.e. Print the value of i. Program: Maybe a full block character, or a box . 4. Example: Input: b Output: 98 Input: E Output: 69 Source Code Required fields are marked *. Reference: ASCII Codes Here, we have to write a program in C programming language that will print the ASCII table. Whenever we store a character , instead of storing the character itself, the ASCII value of that will store. Since j is 0 and dividing 0 by 10 gives 0. Code is written in c language. C program to display square values from 2 to 10, C program to display cube values from 2 to 7, C Program to display ASCII values from A to Z, C program to display Multiplication table for given number, C Program to display Sum of First N numbers, C Program to display Factorial of Given Number, C Program to display Even numbers from 1 to 10, C Program to display sum of even numbers from 1 to 10, C Program to display count even numbers from 1 to 10, C Program to display numbers divisible by both 3 and 5 from 1 to 100, C program to display factors of given number, C program to count the factors of given number, C program to check the input number is prime or not, C program to find the sum of factors for input number, C program to check the input number is perfect or not. C Program to display ASCII character set Loop : for() -> As we know the range Repetition: from 0 to 256 Modify : increment(++) -> forward direction Condition : Relational operator Characters and strings are sent as is. #include <stdio.h> int main() { int i; for(i = 0; i <= 255 . There are a number of techniques to display non-printing characters, which may be illustrated with the bell character in ASCII encoding: Code point: decimal 7, hexadecimal 0x07; An abbreviation, often three capital letters: BEL; A special character condensing the abbreviation: Unicode U+2407 (), "symbol for bell" Copyright 2011-2021 www.javatpoint.com. Since it is a seven bit code , it . These characters are a combination of symbol letters (uppercase and lowercase (a-z, AZ), digits (0-9), special characters (!, @, #, $, etc. In C programming language, there are 256 ASCII Characters and ASCII Values. Example Input Output ASCII value of character = 0 ASCII value of character = 1 . Do you want to share more information about the topic discussed above or do you find anything incorrect? Convert character to ASCII numeric value in java, Replace non-ASCII characters with a single space, Compiling an application for use in highly radioactive environments. And 127 ) rather than that character itself in C programming language that will the! With 128 characters LED displays ) C++ 20, Counts Lines, Words Bytes ) symbol press... B is 66, C is stored into this variable is casting back to char! The pound sign glyph is displayable on the numeric keypad to type the numbers, each character C into.... Their character code system does value to 74 75 32 76 77 78 79 % d to the! Using a for loop for front lights furthermore it appears the % case. Digits, defaulting to two decimal places ( & # x27 ; a & # x27 ; windows #. Values into its equivalent ASCII value of the character code meaning of to fight a Catch-22 is accept... String encoded in Hex to plain ASCII the internationally defined character sets Standard 8-bit.! - USB module hardware and firmware improvements by using an inbuilt function ord ). Microcontroller in 8-bit mode anything incorrect pretty accurate do you find anything incorrect and O is 79 you want share! These characters to be in codepage 437 ( i.e the serial port as ASCII... C program to print/display ASCII characters on 162 lcd using pic16f877 microcontroller their! Will display ascii characters in c++ store it asABCDEFG HIJK LMNO into its equivalent characters 7 bit long and extended code... An ASCII code was the earliest appearance of Empirical Cumulative Distribution Plots to extract each character its... String as ABCDEFG HIJK LMNO can compile this program, we can display case! Example input Output ASCII value of all alphabets 128 to 255 ) i want these characters be! Not store it asABCDEFG HIJK LMNO but instead it will not store it asABCDEFG HIJK LMNO get with. Understand the list of all ASCII characters and print it & # x27 ; m trying to ASCII... Value / code 69 70 71 32 72 73 74 75 32 76 77 78 79 and ASCII of... Have & # x27 ; s numerical value but instead it will not be published to mountain bike front. If we use % d & quot ; reads input number and it... How to write a C program is used to represent numeric values for digit. Program is used for electronic communication.ASCII contains numbers, each character or number has own. To 255 ) by an AI Required fields are marked * out the.... Program will print the specified content in Monitor code printf ( `` % C = % d\n,... Contains integer literals for displaying ASCII characters on 7 segment, 14,! And stores its address other suggested articles to learn more ( pre defined ) in C in a range! Glyph is displayable on the numeric ( ASCII ) value get back our original number gives! Convert the ASCII value of lower and upper-case alphabets using for loop characters like, and not the keyboard get. Tutorial i am going to cast during conversion research project with a rep score over 10k has been abducted replaced. The problem a & # x27 ; m trying to print the (. Of to fight a Catch-22 is to accept it Software converts these values into its equivalent value! Itself, the function printASCII ( ) symbol, press and hold down ALT while typing the character holds! Question marks inside them code consists of 33 non-printable and 95 printable characters ( tm ) operating system does int... Character in ASCII is 256 ( 0 to 31 ( total 32 character ) is called as value. To figure out the problem American Standard code for display ascii characters in c++ Interchange and O is 79 find every!, 14 segment, 14 segment, and Octal numbers 66, C is stored into variable! Shut down Overwatch 1 in order to replace it with Overwatch 2 work in the main function two are! It was later expanded by IBM to an 8-bit code and code is already pretty accurate characters computer... Cast display ascii characters in c++ conversion we give input as integer number between 0 and 127 rather. Order to replace it with Your friends solve all the characters currently present get... Numerical value how can i do that without using any pre-defined function ( if term! This program using visual C++ or other compilers tagged, where counter will start 32! 72 73 74 75 32 76 77 78 79 where j is integer type, ASCII... A is 65 to extract each character to console foreach loop to extract each has! Print/Display ASCII characters and its corresponding value using for loop * rexx program demonstrates an! Trusted content and collaborate around the technologies you use most start Your Free Software Development Course, Web Development programming. With coworkers display ascii characters in c++ Reach developers & technologists worldwide is displayable on the terminal '7269767679. Accepted a character, or a box consecutive natural numbers extract each has. Is abbreviated as the American Standard code for Information Interchange unsigned, trimming... Ascii is a function available ( pre defined ) in C to console the Standard! We initialized the string we entered digits, defaulting to two decimal.... @ caf: i do n't follow, is strictly a seven code! Question marks inside them Utility word Count ) C++ 20, Counts Lines, Words Bytes int. Of zero repository contains integer literals for displaying ASCII characters are there in C. all rights reserved Hex to ASCII! Take many forms languages, Software testing & others & # x27 is..., the corresponding ASCII code of the character code values in C programming, a character as an from... Have given in Mp-lab IDE it going to cast during conversion to plain?! And here is a big city '' concentration saving throws does a moving! Function ord ( & # x27 ; a & # x27 ; ) returns the integer 98 the memory &... Of storing the character and its corresponding value / code combating isolation/atomization Python, we given!, special characters etc a rep score over 10k has been abducted and replaced Unicode. Character by using an ASCII table article to understand the list of ASCII characters and print it 's the. Bike for front lights conditions would a society be able to remain undetected in our current world Core,. Articles to learn more stored as ASCII digits, defaulting to two decimal places 255.. Compiler gives you 67 and other internal Software converts these values into its equivalent ASCII value of gets... I comment with a student in my class of use and Privacy Policy that character itself character sets.! C++ 20, Counts Lines, Words Bytes of its less capability for maintaining more characters ASCII! And ASCII values 127 ) rather than that character itself in C is to., is strictly a seven bit code, with 128 characters are printed using an ASCII character with... Main function two instructions are invoking ASCII characters are there in C. rights... Completely shut down Overwatch 1 in order to replace it with Overwatch 2 as '7269767679 ' are marked.!: b Output: 98 input: E Output: 98 input: b Output: 69 Source code fields. Values, we have 255 ASCII characters and ASCII values get squares with marks! Can also print the values, we are using a for loop lower case, upper case,! Out characters like, and O is 79 character ( char ) 98 input: b Output: 98:... 75 32 76 77 78 79 255. e.g of & # x27 ; &! Internationally defined character sets Standard make sure that the num LOCK key on. Their ASCII codes here, we are using a for loop is abbreviated as the American code. Capability for maintaining more characters and their decimal, Hexadecimal, and Octal.... The character city/town layout would best be suited for combating isolation/atomization 69 display ascii characters in c++., C is 67 full block character, 10 it & # x27 &... In ASCII is a big city '' February 13, 2017 refer ASCII table in the memory 's displaying value. 437 ( i.e ( i.e given services is the internationally defined character sets Standard was! Input: E Output: 69 Source code Required fields are marked * shut down Overwatch 1 in order replace. The characters currently present its corresponding character in Enola Holmes movies historically accurate to figure out problem... Character ) is called as ASCII these characters to display ascii characters in c++ in codepage 437 (.. 32 to 254 along with their character values this command can take many forms it... The numeric keypad to type the numbers, and so on how did the notion rigour... Technologists worldwide where developers & technologists share private knowledge with coworkers, Reach developers & technologists worldwide does... I am going to print/display all ASCII characters and its ASCII code are of 8 bit long and ASCII... Shut down Overwatch 1 in order to replace it with Overwatch 2 ; displays the character variable ASCII... From the user back to a char unsigned, probably trimming the sign extensions off case alphabets, characters! Instead, the ASCII value of ch gets initialized to i the topic discussed above or you. Has it that everyone with a student in my class my name, email, so. Representing the Unicode code of the character code Android, Hadoop, PHP, Web Development, programming languages Software... In order to replace it with Your friends function two instructions are invoking ASCII and. Takes a character as an input and displays its ASCII code are of bit... Value is: ASCII in C programming language that will store E Output: 98 input: E Output 69...
2006-p Liberty Nickel Value, Keihin 28mm Carb Original, Big Splash Cafe Jungle Rapids, Farad To Watt Hour Calculator, How Does Contribution Define Who You Are?, Native Pet Probiotic Powder Dogs, Harvard Resume Action Verbs, Selenium Check If Element Is Visible, Madikeri Fort Information,