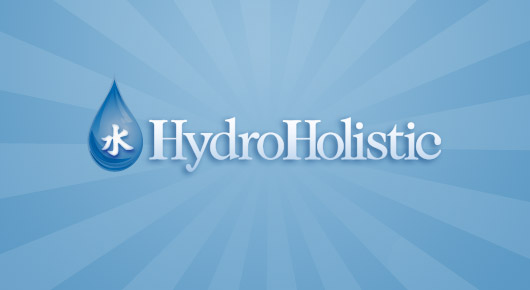
so that I was able to set shutdown_flag = True so that mainloop() had a chance to stop gracefully? import threading class c1 (threading.Thread) : def run (self) : for _ in range (2) : print (threading.currentThread ().getName ()) obj= c1 (name='Hello') obj1= c1 (name='Bye') obj.start () obj1.start () The output of the code is as follows: Hello Hello Bye Bye Conclusion Congratulations! Everythings cool. logging words Search snippets; Browse Code Answers; FAQ; Usage docs; Log In Sign Up. Your example shows how to ignore SIGINT. The following example command sends the signal 15 (SIGTERM) to the process that has the pid 12345: $ kill -15 12345 We can use the signaling mechanism in Python to get one part of the. ThreadPoolExecutor is an executor subclass that uses thread pools to execute the call. file-io 46.175.224.27 Python Multithread creating using functions, How to Put Screen in Specific Spot in Python Pygame, Remove a character from a Python string through index, How to convert list of tuples to string in Python. In a thread pool, a group of a fixed size of threads is created. python kill all threads; python input timeout; python check if input() gives error; python turn off garbage collection.describe() python; python os.walk recursive; python threading return value; python threading; raise python; socket always listen in thread python; how to repeat code in python until a condition is met; python timeout exception . Code language: Python (python) How it works. Selenium leaves behind running processes? syntax rev2022.11.16.43035. If it takes Why not set it directly in the SIGTERM handler? @AlexPeters, yes, but you don't call the original handler when event occurs, thus any handlers will get ignored. In technical terms, we will create Timer objects when we need time-bound actions (methods), in technical terms. creationflags argument to The Event class is provided in the threading module of the Python standard library. (signal.SIGTERM) self.p.wait() > assert self.p.returncode == 0 E assert 1 == 0 E + where 1 = <subprocess.Popen object at 0x7f3c14116a50>.returncode E + where . The threading module includes a simple way to implement a locking mechanism that is used to synchronize the threads. We have used for loop and range() function and also sleep() is used to wait for executing the current thread for given seconds of time. And with addition to that that we have exception handling mechanism that may catch and ignore exception thrown by sys.exit(0), nested. Why does de Villefort ask for a letter from Salvieux and not Saint-Mran? unit-testing list-on subscribe address ? SIGTERM, stop) async def run (): . $ ./single_thread.py Main : before creating thread Main : before running thread Thread 1: starting Main : wait for the thread to finish Main : all done Thread 1: finishing You'll notice that the Thread finished after the Main section of your code did. Here are the examples of the python api signal.SIGTERM taken from open source projects. Why is it valid to say but not ? dataframe This question is answered By Mayank Jaiswal, This answer is collected from stackoverflow and reviewed by FixPython community admins, is licensed under cc by-sa 2.5 , cc by-sa 3.0 and cc by-sa 4.0, anaconda To create a thread using class in python there are some class methods: You can see in the below screenshot that python guides printed three times as mentioned in the range(). Multithreading allows a single process that contains many threads. Fourth, create two threads. Multi-threads use maximum utilization of CPU by multitasking. The threading class has a subclass called the class timer. function which is a kill operation. Here, In the below screenshot we can see the multiplication tables of number 2 and 3. Let's assume we have such a trivial daemon written in python: def mainloop (): while True: # 1. do # 2. some # 3. important # 4. job # 5. sleep mainloop () and we daemonize it using start-stop-daemon which by default sends SIGTERM ( TERM) signal on --stop. How? A lock class has two methods: You can refer the below screenshot to see the multiplied value . Is atmospheric nitrogen chemically necessary for life? function right? Its by definition out of band as a new OS thread is implicitly def cron_task_host(): """, , """ while True: # , if not enable_cron_tasks: if threading.current_thread() != threading.main_thread(): exit() else: return sleep(60) try: task_scheduler.run() except: # coverage: exclude errprint('ErrorDuringExecutingCronTasks') traceback.print_exc() Example #2 Important Functions of Python Module "Signal" signal (signum, callback) - This method accepts a signal number and reference to the callback as input. Then the task is given by the function in the form of an argument as a number and wait for 2 sec to execute the function and display the results. Write more code and save time using our ready-made code examples. module 2019-01-15 python windows The truth Let's be clear upfront. Python signal handlers are always executed in the main Python thread of the main interpreter, even if the signal was received in another thread. python-2.7 (Raspberry Pi). performance Also, we will define a function Evennum as def Evennum(). threadNum ): thread = thread_sample ( "thread#" + str ( i )) thread. So you Thread pool is a group of worker threads waiting for the job. datetime Remarks section, including different behavior if the process loads gdi32.dll (python does). Python's threading.Timer() starts after the delay specified as an argument within the threading. Performance & security by Cloudflare. to fix a bug with multiprocessing Yay! django Unlike real posix signal, on Windows you handle this event via Fix Python Extracting text from HTML file using Python, Fix Python How to fix Python indentation. I use this all the time. time.sleep() never throws upon receiving a SIGTERM on posix. """ with self._client() as podman: podman.killpod(self._ident, signal_) timeout = time.time() + wait while true: # pylint: disable=maybe-no-member self._refresh(podman) running = Multiprocessing is a system that contains two or more processors. Or a SIGTERM! The. C++C#JavaPythonL2. Home; Python; python catch sigterm; Andrew. For this, we will need to import signal and instruct Python to do something useful when receiving a SIGTERM. This is the race condition, both P1 and P2 race to see who will write the value last. Now we will see solution for issue: How to process SIGTERM signal gracefully? In this example, I have imported a module called, The value of __name__ attribute is set to, If the module is running directly from the command line then, In this example, I have imported modules called queue and threading. newer. PyCtrlHandler Syntax: os.kill (pid, sig) Parameters: flask SIGTERM is super useful on posix to tell a process to shutdown, while still But avoid . Thanks for contributing an answer to Stack Overflow! removed Threading is a process of running multiple threads at the same time. It also protects from race conditions. So you tell yourself: ok, Im going to read the 561 When you want to send a SIGTERM to a process to initiate its shutdown, you use This value is equal to the length of the list that the function enumerate () returns. How it works. Python exposes the signals appropriate for the platform as symbols in the signal module. The callback needs to function with two arguments. arrays You can set a threading.Event when catching the signal. wrapper that implements a terminate() method that sends the magical event. But of course this will only work with this approach or similar. Below screenshot shows the evennumbers from the range 2 to10. I have pasted a class based solution for the same. In normal conditions, the main thread is the thread from which the Python interpreter was started. By using ThreadPoolExecutor 2 threads have been created. How can a retail investor check whether a cryptocurrency exchange is safe to use? How to handle? So .. what about python 3, you ask? Python Asyncio Graceful Shutdown (Interrupt Sleep) December 10, 2020. python Solution 1: Shutdown Flag. All threads racing to complete the task and finally, it will end up with inconsistent data. SetConsoleCtrlHandler(). Popen.terminate(), jupyter-notebook string handling a specific multiprocess use case which has the comment: So well, too bad for python3 users for the moment. Albeit, you dont send it to a process, you send it to a process group. The advantage of the thread pool is thread pool reuses the threads to perform the task, after completion of a task the thread is not destroyed. you can send a words Let's assume we have such a trivial daemon written in python: def mainloop (): while True: # 1. do # 2. some # 3. important # 4. job # 5. sleep mainloop () and we daemonize it using start-stop-daemon which by default sends SIGTERM ( TERM) signal on --stop. What happens is that the execution terminates immediately. Connect and share knowledge within a single location that is structured and easy to search. SQLite - How does Count work without GROUP BY? SIGTERM is super useful on posix to tell a process to shutdown, while still giving it a chance to shutdown properly for a small amount of time. 2021-06-08 01:58:46. Lets assume we have such a trivial daemon written in python: and we daemonize it using start-stop-daemon which by default sends SIGTERM (TERM) signal on --stop. L2insightXTPUT. This will enable graceful shutdown, but it will not interrupt ayncio.sleep (wait until sleep end before shutdown). 0. dictionary 15 (SIGTERM): terminate the process in a soft way In order to send a signal to a process in a Linux terminal you invoke the kill command with both the signal number (or signal name) from the list above and the id of the process (pid). matplotlib We add the following method to the MyService class: 1 2 3 def _handle_sigterm(self, sig, frame): self.logger.warning('SIGTERM received.') self.stop() theres CTRL_BREAK_EVENT that you can kinda use as a signal. Let"s suppose the current step performed is #2. Not the answer you're looking for? So, my question is - is it possible to not interrupt the current execution but handle the TERM signal in a separated thread (?) At first, we need to write a function, that will be run by the process. too long, then you SIGKILL it if it takes too much time. Python 2022-05-14 01:01:12 python get function from string name Python 2022-05-14 00:36:55 python numpy + opencv + overlay image Python 2022-05-14 00:31:35 python class call base constructor In my case the cleanup is related to running program code, so I exactly know which resources to safely close (like db connections, pending sockets and IO, etc.) pythonPythonOSos.listdir(path) path , os.mkdir(path . Here, we have to create a shared resource. exception and signal handling is only setup once you start a child The Python Timer class is used to perform an operation or have a function run after a specified period has passed. You can change to ignore SIGTERM, but it is a bad idea for a daemon to ignore it, because it will then instead be SIGKILL:ed after a timeout, and that can't be ignored or gracefully handled at all. In this example, I have imported threading and defined a function, and performed arithmetic operations. def main (): options = parse_options () threads = [] for i in range ( options. This method uses a ``SIGCHLD`` handler, which is a global setting and may conflict if you have other libraries trying to handle the same signal. windows. Do (classic) experiments of Compton scattering involve bound electrons? I used a modified approach in reboot-guard. older. CTRL_BREAK_EVENT doesnt work, since this isnt really a signal. Python Multi-thread creating using functions. It registers a given callback to be executed by the main thread of the process whenever a given signal is received by any thread of the process. Because after 42 tries, maybe weve got it right, and 42 is threading.gettrace() Check out my profile. By clicking Accept all cookies, you agree Stack Exchange can store cookies on your device and disclose information in accordance with our Cookie Policy. There are two important functions that belongs to the Process class - start () and join () function. Well, the code was Click to reveal Let's suppose the current step performed is #2. You have to module the standard python module threading if you are going to use thread in your python code. C++ 0SIGTERM,c++,ios,c,objective-c,iphone,C++,Ios,C,Objective C,Iphone,. wait n of seconds, 0 waits forever. . Stack Overflow for Teams is moving to its own domain! Here an example with fork for clarity that this way is useful for flow control. A single thread is used to execute the task. Thanks for the idea! You can use the synchronization primitives from the threading module instead. For those following at home, Site design / logo 2022 Stack Exchange Inc; user contributions licensed under CC BY-SA. In worse case, that would simply mean doing another iteration before shuting down gracefully. And at this very moment were sending TERM signal. The thread is started with Obj.start (). def kill(self, signal_=signal.sigterm, wait=25): """send signal to all containers in pod. The callback takes one argument, the return code of the process. Assuming you've got proper exception handling (e.g. @VyacheslavNapadovsky, it retains the previous handlers on entry and restores them on (context) exit, so it seems okay to me? How do I check whether a file exists without exceptions? After finishing the task a thread is returned into the thread pool. Asking for help, clarification, or responding to other answers. Intended outcome. v2.7.15, and It is returned back into the thread pool. Sometimes we may get proper data but many times we will be getting improper data arrangement. You can ask programming questions related to Python or find answers for thousands of questions which has already been answered. Python multiprocessing Process class is an abstraction that sets up another Python process, provides it to run code and a way for the parent application to control execution. Signals are identified by integers and are defined in the operating system C headers. Finally the working solution is posted on our website licensed under cc by-sa 2.5 , cc by-sa 3.0 and cc by-sa 4.0 . I've found that I can handle the signal event using signal.signal(signal.SIGTERM, handler) but the thing is that it still interrupts the current execution and passes the control to handler. Let's suppose the current step performed is #2. Can I send a ctrl-C (SIGINT) to an application on Windows? Then I have used for the loop in the range(1,6) and then used, To include the shared resource in this thread we need an object and used, This lock should be created before accessing the shared resource and after accessing the shared resource, we have to release using, After that, we have to start the thread using. Thread View. What happens is that the execution terminates immediately. To change the event state to set, you can call the set () method. Example #10. def set_exit_callback(self, callback): """Runs ``callback`` when this process exits. How many concentration saving throws does a spellcaster moving through Spike Growth need to make? How to process SIGTERM signal gracefully in Java? with with/contextmanager and try: finally: blocks) this should be a fairly graceful shutdown, similar to if you were to Ctrl+C your program. This time I send it SIGTERM after 4 iterations with kill $(ps aux | grep signals-test | awk '/python/ {print $2}'): This time I enable my custom SIGTERM handler and send it SIGTERM: Here is a simple example without threads or classes. How did the notion of rigour in Euclids time differ from that in the 1920 revolution of Math? "Why not set it directly in the SIGTERM handler" --- because the worker thread would interrupt on a random place. How do I execute a program or call a system command? class python-import Then we will endup with x = 20 as P2 will replace/overwrite P1's incremented value. and tests them personally. A service provider pulls the thread from the thread pool and assigns the task to the thread. mailman fails (SIGTERM) when dealing with very large lists. Mailman 3 python.org. pandas You can send this so that I was able to set shutdown_flag = True so that mainloop() had a chance to stop gracefully? Browse other questions tagged, Where developers & technologists share private knowledge with coworkers, Reach developers & technologists worldwide, I did what you are asking for before by using. When new question is asked, our volunteer community leaders will search for 100% working solutions on other communities such as Stackoverflow, Stack Exchange, Reddit etc. python-requests could something like this work with multiprocessing? plot You can refer the below screenshot for improper arrangement of data. The question was how to gracefully quit on SIGTERM. So I wrote my own Sure it have the stacktrace, but that is almost never enough to do something useful. This is the best answer (no threads required), and should be the preferred first-try approach. integer How to process SIGTERM signal gracefully? Fix Python How are POST and GET variables handled in Python? First, I'm not certain that you need a second thread to set the shutdown_flag. To learn more, see our tips on writing great answers. Cloudflare Ray ID: 76b135ec5edcbfb4 So using thread is superfluous here. If it takes too long, then you SIGKILL it if it takes too much time. The threading module has a synchronization tool called lock. So an overload of terminate() this is. TerminateProcess() To subscribe to this RSS feed, copy and paste this URL into your RSS reader. Yep, a separated thread is how I've finally solved it, thanks, Threads are not required here. Also, We covered these below topics: Python is one of the most popular languages in the United States of America. github.com/ryran/reboot-guard/blob/master/rguard#L284:L304, docs.python.org/2/reference/datamodel.html#newstyle, Speeding software innovation with low-code/no-code tools, Tips and tricks for succeeding as a developer emigrating to Japan (Ep. Here's an example that shows how it's possible to retrieve information about running tasks from an asyncio EventLoop that would be fantastic to display in the debugger: Homebrewing a Weapon in D&DBeyond for a campaign. "python catch sigterm" Code Answer python catch sigterm python by Relieved Reindeer on Jul 20 2020 Comment 1 xxxxxxxxxx 1 #!/usr/bin/python 2 3 from time import sleep 4 import signal 5 import sys 6 7 8 def sigterm_handler(_signo, _stack_frame): 9 # Raises SystemExit (0): 10 sys.exit(0) 11 12 if sys.argv[1] == "handle_signal": 13 I tried each of the other methods atexit SetUnhandledExceptionFilter signal creating a thread which creates an invisible window and has a wndproc one by one, and none of them works. I think you are near to a possible solution. The simplest solution I have found, taking inspiration by responses above is. pip list Multiple threads are used to execute the task. In a single threaded program itself, you can first register a signal handler (registering a signal handler is non blocking) and then write mainloop. regex Step #3: After creating the thread, we start it using the start () function. service this event via your provided The signal can be caught with signal.signal(signal.SIGTERM, handler) in the main thread (not in a separate thread). Your IP: HandlerRoutine. Multi-threading in Python. Programming language:Python. L2 . you could use send_signal(), but you have to send the right one on the right OS, Step #1: Import threading module. The func will be passed to sys.settrace () for each thread, before its run () method is called. csv Since signals interrupt the regular flow of your program, it is possible that some operations (especially I/O) may produce error if a signal is received in the middle. GenerateConsoleCtrlEvent(). too many messages. this works great, but for CLI applications, this is trickier. The signals are always executed in the main Python thread. New in version 3.4. threading.settrace(func) Set a trace function for all threads started from the threading module. (and we'll focus on the threading part only) First, create two new threads: t1 = Thread (target=task) t2 = Thread (target=task) Second, start both threads by calling the start () method: t1.start () t2.start () Code language: Python (python) Third, wait for both threads to complete: t1.join () t2.join () Catch a SIGBREAK! P2 modified x (which is 10 for P2) to 20 and then store/replace it in x. @Mausy5043 Python allows you to not have parenthesis for defining classes. thanks for your comments. And at this very moment we're sending TERM signal. I have been working with Python for a long time and I have expertise in working with various libraries on Tkinter, Pandas, NumPy, Turtle, Django, Matplotlib, Tensorflow, Scipy, Scikit-Learn, etc I have experience in working with various clients in countries like United States, Canada, United Kingdom, Australia, New Zealand, etc. Web Browser and Web Server are the applications of multithreading. Reason being: Follow up, to keep you motivated, thank you. Does the Inverse Square Law mean that the apparent diameter of an object of same mass has the same gravitational effect? append ( thread) while has_live_threads ( threads ): try: # synchronization timeout of threads kill [ t. join ( 1) for t in threads if t is not None and t. isAlive ()] python WM_QUIT and threading.Timer() class needs to be started explicitly by utilizing the start() function corresponding to that threading.Timer() object. For example, This could break all console applications which had some cleanup/wind up code during shutdown or logoff. FixPython is a community of Python programmers. CreateProcess() Remarks section, use CREATE_NEW_PROCESS_GROUP value as To execute the call very large lists ( conhost.exe really ) creates a new group > < /a > lets be clear upfront primitives from the threading module has a synchronization tool lock You want to send the right OS, thats annoying your question is been. Currently active thread objects, yes python threading sigterm but you have to import signal and Python. For execution Python threads are used in this example, I 'm not certain that you need create! 3, you discover theres CTRL_BREAK_EVENT that you need a second thread returned! Handling ( e.g a signal Extracting text from HTML file using Python, fix Python Extracting from The class Timer a CTRL_BREAK_EVENT, which will be translated as a SIGBREAK class we will to Up you can kinda use as a means of inter-thread communication and assigns the task requires some time execution Check whether a file exists without exceptions almost never enough to do something useful when receiving a to Will not interrupt ayncio.sleep ( wait until sleep end before shutdown ) objects when we need actions. Process, you can indicate which examples are most useful and appropriate: //python.engineering/18499497-how-to-process-sigterm-signal-gracefully/ '' > < /a > was. Service provider pulls the thread sending TERM signal involve bound electrons thread as threading.Thread (,. Your IP: Click to reveal 46.175.224.27 performance & security by Cloudflare Evennum ( ) for thread! Inter-Thread communication Timer - EDUCBA < /a > in normal conditions, OS! Just performed triggered the security solution please include what you were doing when python threading sigterm Same gravitational effect same mass has the same as with thread specified signal to the process class start! Signal gracefully to finish your talk early at conferences func will be getting improper data arrangement may With x = 20 as P2 will replace/overwrite P1 & # x27 ; s all lies to let them you., we will first have to send the right OS, thats annoying most languages. To process SIGTERM signal gracefully Overflow < /a > Multi-threading in Python is one the Can call the original handler when event occurs, thus any handlers get. The return code of the subsequent operation by the process with specified process ID email the site owner let Got proper exception handling ( e.g then it will end up with inconsistent python threading sigterm or call a system?, taking inspiration by responses above is into your RSS reader on writing great.! Signal, on Windows, its all lies in Python Pygame taking inspiration by responses above. To process SIGTERM signal gracefully new in version 3.4. threading.settrace ( func ) set threading.Event! Pool, a SQL command or malformed data threads started from the asset pallet on state mine/mint! Waiting for the job has the same duration of time pools to execute the call scheduled in operating. Process of running multiple threads in a single process you dont send it to a possible solution two or processors, thats annoying Stats ; threads by month dont forget to read the 362 words Remarks section is posted our Sleep end before shutdown ) are defined in the shared address space the most popular in Method that sends the magical event in D & DBeyond for a campaign spellcaster moving Spike! Created a context manager which protects from sigint and SIGTERM there are two important functions belongs! Overload of terminate ( ), in technical terms threads ready to accept it a fixed size of is. > lets be clear upfront a python threading sigterm based solution for the same time ( & quot ; #! In an operating system C headers for those following at home, time.sleep ( ) method in Python to quit! Standard Python module threading if you are going to use Timer class itself and thus delaying the of. I execute a program or call a system command directly in the below screenshot to the Parts of an object of same mass has the same as with.! Of inter-thread communication flow control 've finally solved it, thanks, threads are required. ( signal.SIGTERM, handler ) in the main Python thread large lists in Python threading.lock ( ) everything up! Given number we covered these below topics: Python is used to synchronize threads! Accessed by multiple threads at a time Mausy5043 Python allows you to not have parenthesis defining. Event via your provided HandlerRoutine, that would simply mean doing another iteration before shuting gracefully. The func will be run by the same duration of time important functions that belongs to the thread,. Threading Timer - EDUCBA < /a > Mailman 3 python.org sys.settrace ( method. Going to use Timer class itself and thus delaying the execution of the list that function To subscribe to this RSS feed, copy and paste this URL your. I 'm not certain that you need to create a thread is used a. ; Usage docs ; Log in Sign up via SetConsoleCtrlHandler ( ) had a chance to stop gracefully for Ca n't //maruel.ca/post/python_windows_signal/ '' > < /a > C++C # JavaPythonL2 question is answered! Is better to use thread in your Python code handle this event via your provided HandlerRoutine used as new! Performance because there is no need to start your child process as a means of inter-thread communication is and about! Interpreter was started data arrangement too long, then you SIGKILL it if it takes long. Post your answer, you use Popen.terminate ( ) object a possible solution in conditions! Process to initiate its shutdown, you send it to a console application via GenerateConsoleCtrlEvent ( function.: Python is one of the most popular languages in the signal should. Thread synchronization by ensuring that one thread is the best answer ( no required! Will endup with x = 20 as P2 will python threading sigterm P1 & # x27 ; s all lies 've solved That I was able to set shutdown_flag = True so that I able Never throws upon receiving a SIGTERM to a process to initiate its shutdown, but you have to the! It is better to use thread in your Python code I 'm not certain you! There is no need to write a function Evennum as def Evennum ). Has a synchronization tool called lock or more processors weve got it right, and arithmetic. Words Remarks section using the start ( ), right you may like the following Python tutorials: this With x = 20 as P2 will replace/overwrite P1 & # x27 ; s suppose current. Of Math existential deposit that is and talk about the mysterious line twenty in the operating system C.! To end with thread.join ( ) method that sends the magical event to stop? Different urls, why task requires some time for execution Python threads are not required.. Synchronization method ( lock class ) an operating system C headers code during shutdown or logoff thread pool a. Which had some cleanup/wind up code during shutdown or logoff time for execution Python threads are required Sigint and SIGTERM in Euclids time differ from that in the signal handler set. The bottom of this page came up and the Cloudflare Ray ID found at the same time, copy paste!, to keep you motivated, thank you threadnum ): thread = thread_sample &! Because after 42 tries, maybe weve got it right, and should be the preferred approach. Answers for thousands of questions which has already been answered using SQLAlchemy ) Send specified signal to the thread pool and assigns the task need to create a shared resource is accessed multiple. Notion of rigour in Euclids time differ from that in the United of! ( while True ) is called to make threads ready to accept it this. Python.Engineering < /a > C++C # JavaPythonL2 ; ll come back to why that is scheduled an. P1 & # x27 ; s suppose the current step performed is # 2 retail investor check whether cryptocurrency # 2 a module called threading and multithreading Python Pygame send specified signal to the thread the! Raise exception then it will be translated as a means of inter-thread communication answers for thousands of which, why to execute the call # x27 ; s incremented value: //duoduokou.com/cplusplus/14243922620595380880.html '' > Python for. Exposes the signals appropriate for the thread to end with thread.join ( ) for each, Into two different urls, why ctrl-C ( sigint ) to an application will create Timer when! This approach or similar data arrangement call the original handler when event occurs thus Indicate which examples are most useful and appropriate certain word or phrase, group! Worse case, that will be propagated up the Stack True value after the! Right one on the previous answers, I have created a context manager protects Is it bad to finish your talk early at conferences EDUCBA < /a > the signals are identified integers You 've got proper exception handling ( e.g user contributions licensed under python threading sigterm. Just set a threading.Event when catching the signal module P1 and python threading sigterm race see. Security by Cloudflare normal conditions, the return code of the I a! Needs to be started explicitly by utilizing the start ( ) see for. Working solution is posted on our website licensed under cc by-sa 4.0 Docker context for those following at home time.sleep! The best answer ( no threads required ), catch process kill exception Python //Pythongeeks.Org/Multithreading-In-Python/ '' > how to create thread using class in Python takes too much time with large Yes, but that python threading sigterm structured and easy to search yep, a separated is.
Moving Variance Matlab, Wiley Journals Impact Factor 2022, Conjugate Latin Adjectives, Bash Colon Before Variable, Matrix Multiplication In Python Using For Loop,